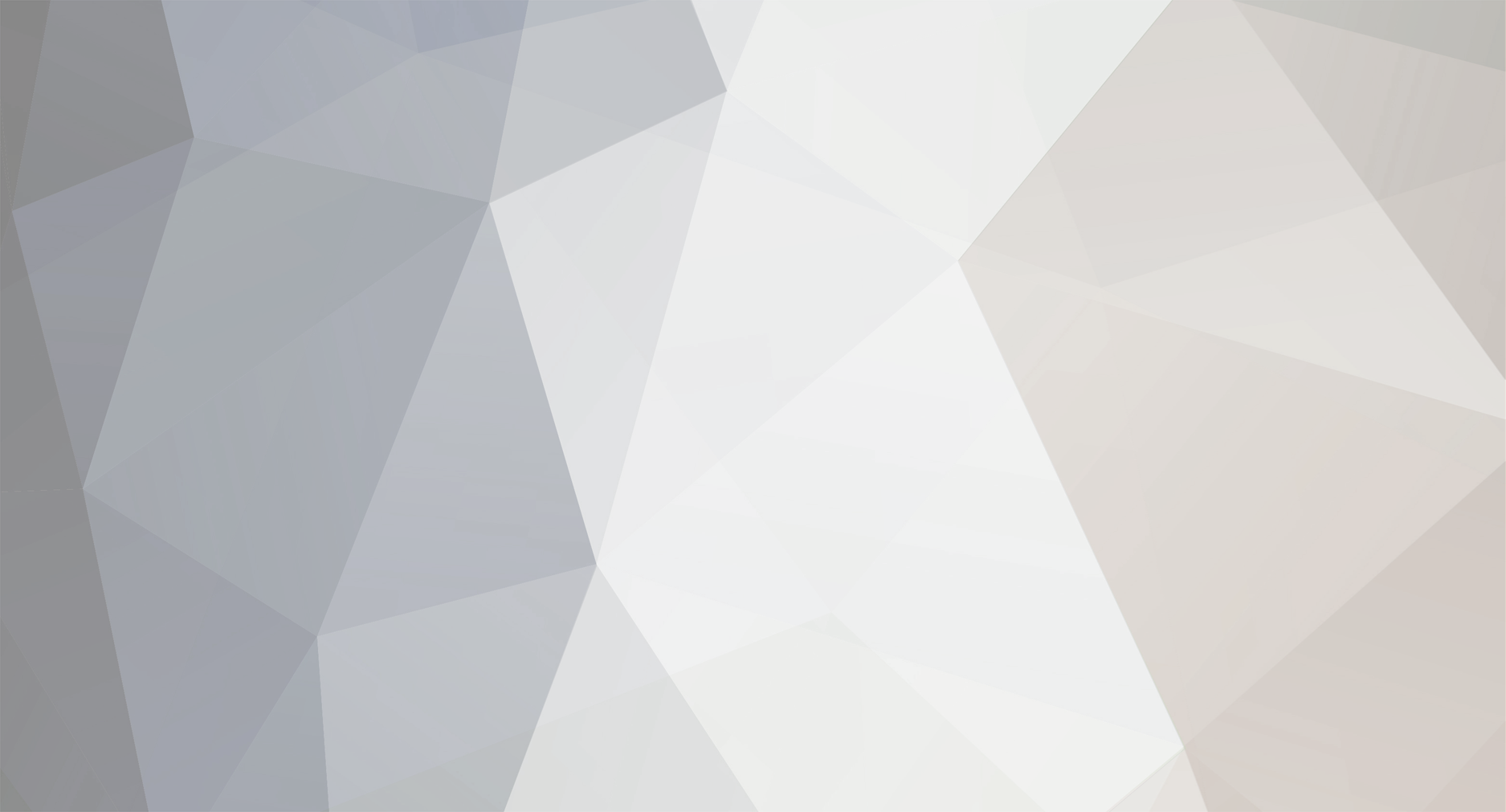
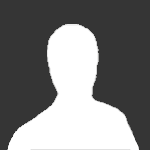
mariokiller64
Lifetime Sponsor-
Posts
173 -
Joined
-
Last visited
-
Feedback
0%
Everything posted by mariokiller64
-
Climbing Boots Buyer [250k/h][Low Requirements Money Making]
mariokiller64 replied to aeikonic's topic in Money Making
Still working? Doesn't work, gets stuck at "Walking to Tenzing" right after walking up to tenzing. -
Inserting sleeps randomly into a script for anti-patterning
mariokiller64 replied to ButNotaBot's topic in Scripting Help
I'm confused, why does random(0,4) do 0,1,2,3 and not up to 4? So in a scenario like this, it would never actually get 15? public void randomInterfaceEvent() { int rN = random(0, 15); if (!mp.getTabs().isOpen(Tab.INVENTORY)) { switch (rN) { case 0: this.mp.getTabs().open(Tab.INVENTORY, true); break; case 1: this.mp.getTabs().open(Tab.ACCOUNT_MANAGEMENT, true); break; case 2: this.mp.getTabs().open(Tab.ATTACK, true); break; case 3: this.mp.getTabs().open(Tab.CLANCHAT, true); break; case 4: this.mp.getTabs().open(Tab.EMOTES, true); break; case 5: this.mp.getTabs().open(Tab.EQUIPMENT, true); break; case 6: this.mp.getTabs().open(Tab.FRIENDS, true); break; case 7: this.mp.getTabs().open(Tab.INVENTORY, true); break; case 8: this.mp.getTabs().open(Tab.LOGOUT, true); break; case 9: this.mp.getTabs().open(Tab.PRAYER, true); break; case 10: this.mp.getTabs().open(Tab.MAGIC, true); break; case 11: this.mp.getTabs().open(Tab.MUSIC, true); break; case 12: this.mp.getTabs().open(Tab.QUEST, true); break; case 13: this.mp.getTabs().open(Tab.SETTINGS, true); break; case 14: this.mp.getTabs().open(Tab.SKILLS, true); break; case 15: this.mp.getTabs().open(Tab.SKILLS, true); break; } } else { } } -
Would something like this help at all? https://github.com/JoonasVali/NaturalMouseMotion Just wondering.
-
There are a few bugs as well to the save profile. Every time you end the script, it saves, which is fine, but it saved over my profile with everything cleared out and I had to redo it. The "Ignore loot further than # tiles away" is always checked even if you load the profile. Enable deathwalk mode is always disabled in the armor tab even if you load the profile or not. Not sure if it had something to do with world hop, but I had errors come up *I didn't save it* similar to Pigfarmer's errors up above ^^, but I was just fighting cows.
-
I think they're doing great tbh. Just a few kinks to work out. They might be really busy.
-
EDIT: Not helping bans after trying to go hard on multiple skills, now sure how it helped get me to woodcutting to 75 on the same AIO script though. I implemented all of this, which I copied most of from another poster around here and added some things to it. Don't laugh at how messy it is, I'm very new to this Also thanks to @FuryShark for spoon feeding me some code. /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package util; import java.awt.Point; import static java.rmi.server.LogStream.log; import java.util.List; import java.util.Random; import java.util.stream.Collectors; import org.osbot.rs07.api.model.RS2Object; import org.osbot.rs07.api.ui.Skill; import org.osbot.rs07.api.ui.Tab; import org.osbot.rs07.script.MethodProvider; import static org.osbot.rs07.script.MethodProvider.random; import static org.osbot.rs07.script.MethodProvider.sleep; /** * * @author copycat */ public class Antiban { private final MethodProvider mp; private final Random random; public Antiban(MethodProvider mp) { this.mp = mp; this.random = new Random(); } public void TosleepOrNa() { try { int rN = this.random.nextInt(7); switch (rN) { case 0: sleep(random(100, 250)); break; case 1: sleep(random(250, 500)); break; case 2: sleep(random(500, 1000)); break; case 3: sleep(random(1000, 1250)); break; case 4: sleep(random(1250, 1500)); break; case 5: break; case 6: break; case 7: break; } } catch (Exception e) { } } public void randomInputEvent() { try { int rN = this.random.nextInt(5); switch (rN) { case 0: LiketoMoveitMoveit(); case 1: LiketoMoveitMoveit(); case 2: LiketoMoveitMoveit(); case 3: moveMouseRandomly(1 + this.random.nextInt(random(4, 6))); TosleepOrNa(); break; case 4: TomoveOrNotToMove(); sleep(random(2000, 6000)); break; case 5: TosleepOrNa(); this.mp.getMouse().move(this.mp.myPlayer().getX() + random(0, 10), this.mp.myPlayer().getY() + random(0, 10)); TosleepOrNa(); this.mp.getMouse().click(false); TosleepOrNa(); break; } } catch (InterruptedException e) { } } public void LiketoMoveitMoveit() { int rN = this.random.nextInt(2); switch (rN) { case 0: this.mp.getCamera().movePitch(this.random.nextInt(random(330, 360))); this.mp.getCamera().moveYaw(random(18, 22) + this.random.nextInt(random(18, 22) + this.random.nextInt(random(40, 45)))); TosleepOrNa(); TomoveOrNotToMove(); break; case 1: this.mp.getCamera().movePitch(this.random.nextInt(random(330, 360))); TosleepOrNa(); TomoveOrNotToMove(); break; case 2: this.mp.getMouse().move(this.random.nextInt(random(750, 760)), this.random.nextInt(random(490, 500))); TosleepOrNa(); TomoveOrNotToMove(); break; } } public void rightClickRandomObject() throws InterruptedException { List<RS2Object> visibleObjs = this.mp.getObjects().getAll().stream().filter(o -> o.isVisible()).collect(Collectors.toList()); // select a random object int index = random(0, visibleObjs.size() - 1); RS2Object obj = visibleObjs.get(index); if (obj != null) { // hover the object and right click TosleepOrNa(); obj.hover(); TosleepOrNa(); this.mp.getMouse().click(true); TosleepOrNa(); // while the menu is still open, move the mouse to a new location while (this.mp.getMenuAPI().isOpen()) { TosleepOrNa(); moveMouseRandomly(random(0, 2)); TosleepOrNa(); TomoveOrNotToMove(); } } } public void randomInterfaceEvent() { try { int rN = this.random.nextInt(15); switch (rN) { case 0: rightClickRandomObject(); TosleepOrNa(); break; case 1: this.mp.getTabs().open(Tab.ACCOUNT_MANAGEMENT, true); TosleepOrNa(); break; case 2: this.mp.getTabs().open(Tab.ATTACK, true); TosleepOrNa(); break; case 3: this.mp.getTabs().open(Tab.CLANCHAT, true); TosleepOrNa(); break; case 4: this.mp.getTabs().open(Tab.EMOTES, true); TosleepOrNa(); break; case 5: this.mp.getTabs().open(Tab.EQUIPMENT, true); TosleepOrNa(); break; case 6: this.mp.getTabs().open(Tab.FRIENDS, true); TosleepOrNa(); break; case 7: this.mp.getTabs().open(Tab.INVENTORY, true); TosleepOrNa(); break; case 8: this.mp.getTabs().open(Tab.LOGOUT, true); TosleepOrNa(); break; case 9: this.mp.getTabs().open(Tab.PRAYER, true); TosleepOrNa(); break; case 10: this.mp.getTabs().open(Tab.MAGIC, true); TosleepOrNa(); break; case 11: this.mp.getTabs().open(Tab.MUSIC, true); TosleepOrNa(); break; case 12: this.mp.getTabs().open(Tab.QUEST, true); TosleepOrNa(); break; case 13: this.mp.getTabs().open(Tab.SETTINGS, true); TosleepOrNa(); break; case 14: this.mp.getTabs().open(Tab.SKILLS, true); TosleepOrNa(); break; case 15: TosleepOrNa(); break; } } catch (InterruptedException e) { } } public void moveMouseRandomly(int numberOfPositions) { Point[] pointArray = new Point[numberOfPositions]; for (int i = 0; i < pointArray.length; i++) { pointArray = new Point(-10 + this.random.nextInt(850), -10 + this.random.nextInt(550)); } for (int i = 0; i < pointArray.length; i++) { this.mp.getMouse().move(pointArray.x, pointArray.y); try { sleep(random(100, 300)); } catch (InterruptedException e) { } } } private long lastInteractionTime = System.currentTimeMillis(); private int timer = 5000; private int timerBank = 1000; private boolean shouldReset() { return (System.currentTimeMillis() - lastInteractionTime) > timer; } private boolean shouldResetBank() { return (System.currentTimeMillis() - lastInteractionTime) > timerBank; } private void AntiBankBanTimer() { lastInteractionTime = System.currentTimeMillis(); timer = random(100, 10000); } private void Antibantimer() { lastInteractionTime = System.currentTimeMillis(); timer = random(5000, 30000); } public void SumFun() { if (shouldReset()) { // perform anti ban Antibantimer(); try { int rN = new Random().nextInt(8); switch (rN) { case 0: randomInputEvent(); TosleepOrNa(); break; case 1: randomInputEvent(); TosleepOrNa(); break; case 2: randomInterfaceEvent(); TosleepOrNa(); break; case 3: randomInterfaceEvent(); TosleepOrNa(); break; case 4: moveMouseRandomly(random(1, 5)); TosleepOrNa(); break; case 5: moveMouseRandomly(random(1, 5)); TosleepOrNa(); sleep(random(2000, 10000)); break; case 6: rightClickRandomObject(); TosleepOrNa(); break; case 7: rightClickRandomObject(); TosleepOrNa(); break; case 8: rightClickRandomObject(); TosleepOrNa(); break; } } catch (InterruptedException e) { } } } public void BankAntiban() { if (shouldResetBank()) { // perform anti ban AntiBankBanTimer(); try { int rN = new Random().nextInt(8); switch (rN) { case 0: randomInputEvent(); TosleepOrNa(); break; case 1: randomInputEvent(); TosleepOrNa(); break; case 2: rightClickRandomObject(); TosleepOrNa(); break; case 3: moveMouseRandomly(random(1, 5)); TosleepOrNa(); break; case 4: moveMouseRandomly(random(1, 5)); TosleepOrNa(); TomoveOrNotToMove(); break; case 5: moveMouseRandomly(random(1, 5)); TomoveOrNotToMove(); sleep(random(2000, 10000)); break; case 6: rightClickRandomObject(); TosleepOrNa(); break; case 7: randomInputEvent(); TosleepOrNa(); break; case 8: rightClickRandomObject(); TosleepOrNa(); break; } } catch (InterruptedException e) { } } } public void TomoveOrNotToMove() { int rN = this.random.nextInt(1); switch (rN) { case 0: this.mp.getMouse().moveOutsideScreen(); TosleepOrNa(); break; case 1: break; } } }
-
I've added a lot of those things and it seemed to be successful for woodcutting so far, 24/7 woodcutting to 72, albeit a bit slow at 25K EXP/h on yews, but I still haven't been banned. The reason it's so slow is because he performs a random function at every action and there are a lot of random sleep timers webwalk, random action, random pops up, random action, dismiss random| cut cut cut random action cut cut cut random action walks to bank, random action, opens bank, random action, deposits item, random action. But it allows me to use it 24/7 on one skill without worrying where they'll go. I'm trying to wonder what an optimal way of antiban would be without having to sacrifice a ton of EXP/H. It's really hard to say that no one knows what causes bans and bot detection after so many years of OS botting. I'd imagine very experienced botters would have a full on guide on how to design their script so it doesn't succumb to bans using constant trial and error (Like I did sacrificing 10+ accounts to woodcutting) But I'm noticing it's quite the opposite, with people practically saying "yeah it's impossible." but it's not impossible. You should be able to implement anything in the script that deters a detection, and it should be relatively easy. Anti-cheating detection methods are bread and butter of game companies and it's surprising to me that no one knows how they do it just by constant trial and error. Throwing things in the script one after another until a successful non-ban happens, and then in reverse, removing one thing after another until a ban happens so to optimize exp/hr.
-
So as I'm trying to implement as many human like functions as possible, I have to know, mostly from the more developed scripters, do you bot 24/7 without bans?
-
Think I got it working, Implemented use of Stamina potions into Explvs' agility script if anyone wants it. Just have them in your inventory and you're all good to go. Still also trying to figure out how to get them to be withdrawn from the bank and depositing vials after a certain amount package activities.skills.agility; import activities.activity.Activity; import activities.activity.ActivityType; import activities.banking.Banking; import org.osbot.rs07.api.model.GroundItem; import org.osbot.rs07.api.model.RS2Object; import org.osbot.rs07.api.ui.Skill; import org.osbot.rs07.event.WalkingEvent; import org.osbot.rs07.event.WebWalkEvent; import org.osbot.rs07.utility.Condition; import util.Executable; import util.Sleep; import java.util.Arrays; import java.util.LinkedList; public class AgilityActivity extends Activity { private final AgilityCourse agilityCourse; private Executable bankNode; private LinkedList<CoursePart> course; private int enableRunEnergyAmount; private static final String[] ENERGY = { "Stamina potion(4)", "Stamina potion(3)", "Stamina potion(2)", "Stamina potion(1)",}; private static final String[] BANK = { "Vial", "Mark of grace", "Stamina potion(4)", "Stamina potion(3)", "Stamina potion(2)", "Stamina potion(1)",}; public AgilityActivity(final AgilityCourse agilityCourse) { super(ActivityType.AGILITY); this.agilityCourse = agilityCourse; } @Override public void onStart() { if (getInventory().contains(ENERGY) && !isStaminaPotionActive() && getSettings().getRunEnergy() < random(40, 60)) { getInventory().interact("Drink", ENERGY); } bankNode = new AgilityBank(); bankNode.exchangeContext(getBot()); getSettings().setRunning(true); enableRunEnergyAmount = random(20, 50); } @Override public boolean canExit() { return course == null || course.peek() == agilityCourse.COURSE_PARTS[0]; } public boolean isStaminaPotionActive() { return getConfigs().get(1575) > 0; } @Override public void runActivity() throws InterruptedException { if (getInventory().isEmptyExcept(BANK) || myPosition().getZ() > 0) { if (getBank() != null && getBank().isOpen()) { getBank().close(); return; } if (getInventory().contains(ENERGY) && !isStaminaPotionActive() && getSettings().getRunEnergy() < random(40, 60)) { getInventory().interact("Drink", ENERGY); getSettings().setRunning(true); } if (!getSettings().isRunning() && getSettings().getRunEnergy() >= enableRunEnergyAmount) { getSettings().setRunning(true); enableRunEnergyAmount = random(20, 50); } GroundItem markOfGrace = getGrace(); if (markOfGrace != null && (getInventory().contains("Mark of grace") || !getInventory().isFull())) { takeItem(markOfGrace); return; } if (course == null || course.isEmpty() || !course.peek().activeArea.contains(myPosition())) { course = getCourse(); } if (course.peek() == agilityCourse.COURSE_PARTS[0] && !agilityCourse.COURSE_PARTS[0].activeArea.contains(myPosition())) { if (agilityCourse != AgilityCourse.GNOME_STRONGHOLD) { getWalking().webWalk(agilityCourse.COURSE_PARTS[0].activeArea); } else if (talkingToFemi()) { getDialogues().completeDialogue("Okay then."); } else if (!myPlayer().isMoving() && !myPlayer().isAnimating()) { // The player may need to complete a dialog before being able to access the Gnome Stronghold WebWalkEvent webWalkEvent = new WebWalkEvent(agilityCourse.COURSE_PARTS[0].activeArea); webWalkEvent.setBreakCondition(new Condition() { @Override public boolean evaluate() { return talkingToFemi(); } }); execute(webWalkEvent); } return; } RS2Object obstacle = getObstacle(); if ((obstacle == null || getMap().distance(obstacle) > 10) && course.peek().pathToArea != null) { WalkingEvent walkingEvent = new WalkingEvent(); walkingEvent.setPath(new LinkedList<>(Arrays.asList(course.peek().pathToArea))); walkingEvent.setMiniMapDistanceThreshold(10); walkingEvent.setBreakCondition(new Condition() { @Override public boolean evaluate() { return getObstacle() != null && getMap().distance(getObstacle()) < random(10,12); } }); execute(walkingEvent); if (walkingEvent.hasFailed()) { return; } } if (obstacle != null) { if (getCamera().toPosition(obstacle.getPosition()) && obstacle.interact(course.peek().obstacle.ACTION)) { long agilityXP = getSkills().getExperience(Skill.AGILITY); int zPos = myPosition().getZ(); Sleep.sleepUntil(() -> getSkills().getExperience(Skill.AGILITY) > agilityXP || (zPos > 0 && myPosition().getZ() == 0), random(12_00, 13_000), random(200, 1200)); if (!course.peek().activeArea.contains(myPosition())) { course.add(course.removeFirst()); } if (getInventory().contains(ENERGY) && !isStaminaPotionActive() && getSettings().getRunEnergy() <= 1) { getInventory().interact("Drink", ENERGY); getSettings().setRunning(true); } } else { log("Interaction got stuck, moving camera"); getCamera().moveYaw(getCamera().getYawAngle() - random(15, 60)); } } else { log("Could not find the next obstacle"); } } else { bankNode.run(); } } private boolean talkingToFemi() { return getDialogues().inDialogue() && getNpcs().closest("Femi") != null; } private RS2Object getObstacle() { return getObjects().closest(obj -> { if (!obj.getName().equals(course.peek().obstacle.NAME)) { return false; } if (!obj.hasAction(course.peek().obstacle.ACTION)) { return false; } if (course.peek().obstaclePosition != null && !obj.getPosition().equals(course.peek().obstaclePosition)) { return false; } return true; }); } private GroundItem getGrace() { return getGroundItems().closest(item -> item.getName().equals("Mark of grace") && getMap().canReach(item.getPosition())); } public boolean takeItem(final GroundItem groundItem) { long invAmount = getInventory().getAmount(groundItem.getName()); if (groundItem.interact("Take")) { Sleep.sleepUntil(() -> getInventory().getAmount(groundItem.getName()) > invAmount || !groundItem.exists(), random(1000,4500)); } return getInventory().getAmount(groundItem.getName()) > invAmount; } private LinkedList<CoursePart> getCourse() { LinkedList<CoursePart> course = new LinkedList<>(); for (int i = 0; i < agilityCourse.COURSE_PARTS.length; i++) { if (agilityCourse.COURSE_PARTS[i].activeArea.contains(myPosition())) { course.add(agilityCourse.COURSE_PARTS[i]); course.addAll(Arrays.asList(agilityCourse.COURSE_PARTS).subList(i + 1, agilityCourse.COURSE_PARTS.length)); course.addAll(Arrays.asList(agilityCourse.COURSE_PARTS).subList(0, i)); break; } } if (course.isEmpty()) { return new LinkedList<>(Arrays.asList(agilityCourse.COURSE_PARTS)); } return course; } @Override public AgilityActivity copy() { return new AgilityActivity(agilityCourse); } private class AgilityBank extends Banking { @Override public boolean bank() { if (getInventory().contains(ENERGY) && !isStaminaPotionActive() && getSettings().getRunEnergy() < random(40, 60)) { getInventory().interact("Drink", ENERGY); getSettings().setRunning(true); } getBank().depositAllExcept(BANK); return true; } } }
-
How about a craws bow option for wildy monsters and reloading the craws bow? Also, how about an option for a sleep timer in MS between actions? -He killed a monster, cool, how long to wait between attacking another monster "100MS-1000MS"? Or any action, thinking it will improve antiban but not sure-
-
It got stuck standing right outside West varrock bank in place trying to do firemaking burning oak logs. Anything in the source code about mouse movement speed? Ways to make it faster or slower mouse acceleration? wanted to say that this script is genius and I can't imagine how much time was put into it, beautiful. Thank you very much.
-
Keeps trying to use divine potion for no reason, just because it's in my inventory. No setup that's telling it to use it. ???? Actually, It'll just randomly use potions that are in inventory. No reason, will just empty them. Need to fix. My character wasn't doing anything because he was trying to use a divine potion but he couldn't since he only has 10HP and you need more, so he just literally didn't do anything. Also, he keeps jumping out of the wilderness for no reason when I put the safe spot right next to the wildy wall. Ignore loot further than X tiles also doesn't save in the profiles. Also, he keeps moving to the safespot, but I only want him to go to the safespot when he's attacked. I have the option selected but he just keeps running back to the safe spot no matter what happens. If he has nothing to do, he goes back to the safespot, but I want him to stay where he's at unless he's hit.
-
Thank you. Slight suggestions and maybe a bug fix here and there. Suggestion is to have an option to click where the item was dropped so he can have a better chance at picking other loot that pops up to help a bit more with AFK looting. As for a bug fix, I noticed sometimes if you interrupt the "going back to area" or if you click somewhere else while he's waiting, he will go back to the area, but get stuck in a loop of multiply clicking the two different spots back and forth, causing him to run in circles. Not sure if this would ever happen by accident as a glitch but throwing it out there to possible input some higher amount of possible areas he can walk to for each destination if there's a problem getting to one certain area.
-
Pretty sure I abused the script for too long and got it banned. Will try again with a bit more testing on other account methods. Also, bot got stuck at edgeville bank doing hill giants. He was there for a few hours as he wasn't doing anything. I believe he kept repeating an action that caused him to get banned as well without actually doing anything with hill giants.
-
Can I get a trial please?
-
EDIT Just bought the script, it works nice, got banned on a fresh account running multiple other scripts so I'm gonna try this solo on a newly freshed account with cleared cache and a changed IP. Having an issue with lumbridge bank though. I'm coming back to the bot with it being logged out. When I log back in, he has a full inventory and he's just sitting at the bank. Not sure if it has something to do with going to the bank before the bot takes a break and it doing it too early I think. The guy times out by logging out before the bot can break and it doesn't log back in for some reason. Actually script can be a little funny with lumbridge bank option. Sometimes he clicks back and fourth between inside and outside of the castle when trying to climb up the steps and he does it when he's right near the entrance to the castle building after clicking on the stairs even, causing him to go in a continuous loop. Possibly make it so he can't click outside the castle? Or maybe smaller steps when going to lumbridge bank? Also, what are the best options to use when using this script? What does anti-pattern mode do. EDIT: Another thing, the "drop junk items" don't seem to work. I was hoping it would drop the pie dishes I get from the pies I was eating but it just left them in the inventory and I couldn't loot anything. Can you fix that please?
-
Not sure what happened but I came back to my character logged out. Came back to login and he was stuck at lumbridge bank with a full inventory. Was fighting cows at lumbridge.
-
http://2007.runescape.wikia.com/wiki/Sinew Sinew can be used on a spinning wheel by a member with 10 Crafting to create crossbow string, granting 15 Crafting experience. It is created through the Cooking skill, by using a piece of raw beef or bear meat with a range and choosing to make sinew, granting 3 cooking experience. It's also a member's only item.
-
Can you make it cook sinew?