-
Posts
239 -
Joined
-
Last visited
-
Days Won
1 -
Feedback
100%
yfoo last won the day on April 30 2024
yfoo had the most liked content!
About yfoo
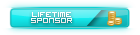
Profile Information
-
Gender
Male
-
Location:
SW U.S
Recent Profile Visitors
4004 profile views
yfoo's Achievements

Steel Poster (4/10)
99
Reputation
-
Unpatch the client. It is done the same way you patched the client.
-
Does activate mean I have to press 1,2,3,4 when the event starts OR is it enable/disable the events the script is allowed to do?
-
I think this is a case where the numpad 1 and the 1 below the f keys are considered to be different.
-
a switch to the c++ client is in the works.
-
Some sequences used by me. You can copy/paste them here instead of making your own. Name the whatever you want BUT they must have the .json file extention. C:\Users\<Your Windows Account>\OSBot\Data\Blast_Miner North East Meta. Same as what is shown on the wiki (https://oldschool.runescape.wiki/w/Blast_mine/Strategies#North-east) North West Meta. (https://oldschool.runescape.wiki/w/Blast_mine/Strategies#North-west_8-rock)
-
yBlastMine Why do it when I can do it for you. This is a preview thread. The script is complete except minor pieces (handle clue geodes, pick up ore from NPC, add prebuilt sequences, update UI) It will be released after Osbot moves to C++ client. New issues may arise when the client is updated. Features Create a unique blast mining sequence exclusive to you. Load multiple sequences, the script will randomly switch between them Failsafe! Multiple consecutive failures will automatically stop the script. Anticrash Auto Random Event + Genie Support Script Setup Documentation 1. On startup, A GUI will appear with the following options. If this is your first run of this script, you will have to create your own sequence. Click the Record Sequence button to proceed. 2. The Osbot painter will highlight positions of interest to blast-mining. For your convivence, clicking highlighted positions will not pass the click to Runescape, meaning you can select positions without moving your character. You can still move by clicking any non-highlighted position. - CYAN WITH NUMBER + GREEN WALLS: Positions you selected, Number indicates their selection order. The highlighted green walls indicate the what will be interacted with at that position. - CYAN: Positions that can be selected. Hovering turns them yellow. Click to select. - YELLOW: The current hovered position. 3. When you are finished, click the Save Sequence button to open a separate window to save your sequence. Sequences are saved in your Osbot folder (C:\Users\<Your Windows Account>\OSBot\Data\Blast_Miner). The files created by this script are openable by any text editor. This is the contents of the file I just saved in this demo; it is a list of positions. ``` [ { "x": 1472, "y": 3870, "z": 0 }, { "x": 1472, "y": 3872, "z": 0 }, { "x": 1475, "y": 3876, "z": 0 }, { "x": 1476, "y": 3879, "z": 0 } ] ``` 4A. When The sequence is saved, the original GUI in Step 1 will reappear. After clicking the Load from JSON button, a file explorer window will allow you to select the newly created sequence. Selecting and opening a file will preview that sequence in the osbot painter. You can now start the script, your character will now follow the sequence you've created. 4B. When selecting sequence files, you can elect to select more than one. The script will randomly select a sequence to execute for a random number of cycles. Afterwards another sequences will be randomly selected. This will repeat for the script's duration. ex: Sequence1 (10 cycles) -> Sequnce3 (15 cycles) -> Sequence2 (13 cycles) -> Sequence1 (17 cycles) -> ...
-
Mirror lags behind a day or 2 unfortunately.
-
Wow really nice. Mind adding a random gaussian sleep after all bars have been smelted before banking?
-
Very nice. Thanks for sharing.
-
Selecting the Closest Battlestaff in crafting script
yfoo replied to timepudding's topic in Scripting Help
Use ```inventory.interact(13,"Use"))``` To select slot 13. followed by ```inventory.interact(14, USE)```. Here is how I dod mine private boolean combineComponents() throws InterruptedException { int[] slotPair = getInvSlotPair(); Item item1 = inventory.getItemInSlot(slotPair[0]); Item item2 = inventory.getItemInSlot(slotPair[1]); boolean notNull = item1 != null && item2 != null; boolean notSameItem = notNull && item1.getId() != item2.getId(); // Assert Item1 and 2 are the equivalent items determined at script start (ItemA and B). // Not foolproof, but a simple method boolean sumTo0 = notNull && (item1.getId() + item2.getId() - itemA.getId() - itemB.getId() == 0); boolean canUseSlotPair = notSameItem && sumTo0; if (canUseSlotPair && inventory.interact(slotPair[0], USE)) { ScriptPaint.setStatus("ItemA -> ItemB"); sleep(randomGaussian(300, 100)); return inventory.isItemSelected() && inventory.interact(slotPair[1], USE); } else { ScriptPaint.setStatus("ItemA -> ItemB w/ backup interaction"); if (inventory.interact(USE, itemA.getId())) { sleep(randomGaussian(300, 100)); return inventory.isItemSelected() && inventory.interact(USE, itemB.getId()); } } return false; } -
When I wrote it like 2 weeks ago it worked. You need to override the break manager with an instance of the class Put this in onStart bot.getRandomExecutor().overrideOSBotRandom(BreakManagerHook.initInstance(bot)); Then use this to start a break early. BreakManagerHook.getInstance().startBreakEarly();
-
Gets stuck on black knights fortress, It attempts to enter through the wrong door, it should go through the single doors next to the guards. https://ibb.co/t3Ghqqb
-
Is VPN subbing for big discounts safe?
yfoo replied to Hernandez2000's topic in Community Discussion
That works sure, but why not just rwt gold then buy bonds. Cheaper than using pesos probably. Buy from a private seller rather than a major site, less chance of being rwt flagged. . -
This was the end result I settled with. I also happened to need a hook to know when the break ended to potentially check if my position was crashed while I was away. The OnExit() allows me to know that. For now I'm not doing anything with it just logging (log("Return from break!");) package Util; import org.osbot.rs07.Bot; import org.osbot.rs07.randoms.BreakManager; public class BreakManagerHook extends BreakManager { private volatile boolean breakEarly; private volatile boolean shouldActivateCalled; private static BreakManagerHook instance; public static BreakManagerHook getInstance() { if(instance == null) { throw new NullPointerException("ExcavatePlantIgnite is null"); } return instance; } public static BreakManagerHook initInstance(Bot bot) { instance = new BreakManagerHook(bot); return instance; } private BreakManagerHook(Bot IiIiiiIiiII) { super(IiIiiiIiiII); } @Override public synchronized boolean shouldActivate() { if(breakEarly && !shouldActivateCalled) { log(String.format("Thread %d notifying...", Thread.currentThread().getId())); shouldActivateCalled = true; this.notify(); } if(super.shouldActivate() && breakEarly) { breakEarly = false; log("breakEarly -> false"); } return super.shouldActivate() || breakEarly; } @Override public void onExit() { super.onExit(); log("Return from break!"); } public synchronized void startBreakEarly() throws InterruptedException { breakEarly = true; shouldActivateCalled = false; while (!shouldActivateCalled) { log(String.format("Thread %d waiting until shouldActivate() called...", Thread.currentThread().getId())); this.wait(); } log("Exited startBreakEarly"); } }