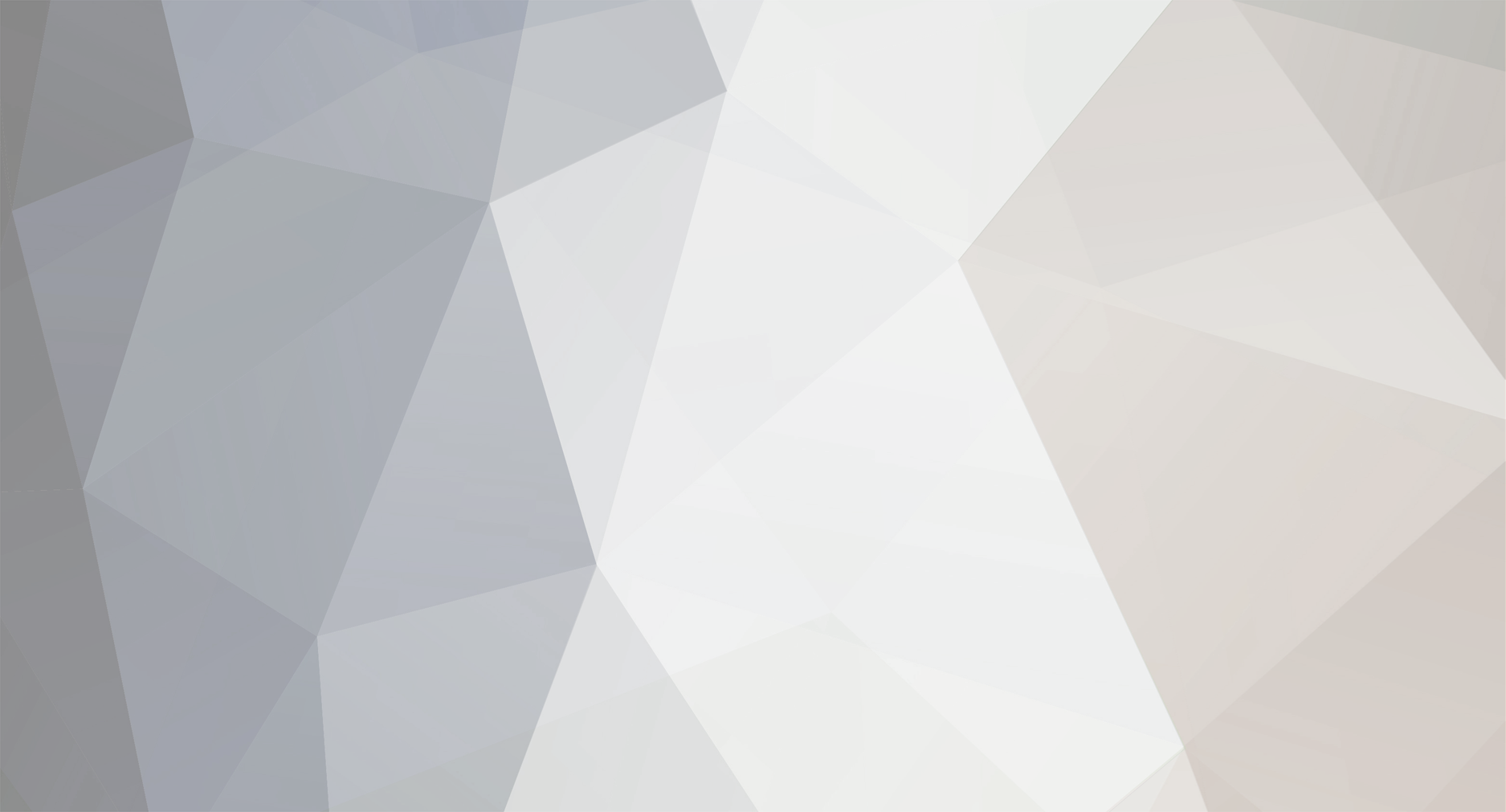
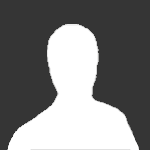
Dextrell
Members-
Posts
16 -
Joined
-
Last visited
-
Feedback
0%
Profile Information
-
Gender
Male
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
Dextrell's Achievements

Newbie (1/10)
2
Reputation
-
Dextrell started following DexCutter - F2P Draynor Woodcutting Script | Woodcutting Guild Mage Trees , DexAlcher and DexCooker
-
Basic Alch script with a small anti ban cheers! Put the item you'd like to alch at the far right in the first row the script will high light a box for you where to place it if you're lost If you want low Alchemy just swap the function call on highAlchemy and it will do that instead! package com.dexalcher; import java.awt.Color; import java.awt.Graphics2D; import org.osbot.rs07.api.ui.RS2Widget; import org.osbot.rs07.api.ui.Skill; import org.osbot.rs07.script.Script; import org.osbot.rs07.script.ScriptManifest; import org.osbot.rs07.utility.ConditionalSleep; @ScriptManifest(name = "DexAlcher", version = 1.0, author = "Dextrell", logo = "", info = "") public class DexAlcher extends Script { private String itemToAlch = "Rune arrow"; public void onStart() throws InterruptedException { getExperienceTracker().start(Skill.MAGIC); } public int onLoop() throws InterruptedException { int rand = random(0, 200); if (rand == 150) { log("moving mouse at random..."); mouse.move(random(0, 2500), random(0, 2500)); } else if (rand == 0) { log("moving mouse off screen..."); mouse.moveOutsideScreen(); } if (canAlch()) { clickedAlch(); } else { stop(); log("Out of supplies or dead"); } return 1000; } public boolean canAlch() { return (getEquipment().isWieldingWeaponThatContains(new String[] { "staff" }) && getInventory().contains(new String[] { "Nature rune", itemToAlch })); } public void clickedAlch() { if (!getInventory().contains(itemToAlch)) { log("We dont have the items stopping..."); stop(); } if (!(getTabs()).magic.open()) { new ConditionalSleep(600, 800) { @Override public boolean condition() throws InterruptedException { return false; } }; } new ConditionalSleep(600, 1000) { @Override public boolean condition() throws InterruptedException { clickHighSpellAlchemy(); return false; } }.sleep(); new ConditionalSleep(600, 1000) { @Override public boolean condition() throws InterruptedException { // final RS2Widget itemPic = getWidgets().get(149, 3); // int clickX = itemPic.getAbsX() + random(0,6); int clickX = random(691, 717); int clickY = random(216, 235); // int clickY = itemPic.getAbsY() + random(0,6); // log("src x: " + itemPic.getAbsX() + " src: " + itemPic.getAbsY()); // log("Click x: " + clickX + " y: " + clickY); mouse.click(clickX, clickY, false); log("Finished clicking item..."); return false; } }.sleep(); } public boolean clickMagicBook() { RS2Widget bookIcon = getWidgets().get(548, 76); int clickX = bookIcon.getAbsX() + random(10, 20); int clikcY = bookIcon.getAbsY() + random(10, 20); return mouse.click(clickX, clikcY, false); } public void clickLowSpellAlchemy() { // RS2Widget alchemyIcon = getWidgets().get(218, 21); // int clickX = alchemyIcon.getAbsX() + random(0,5); // int clickY = alchemyIcon.getAbsY() + random(0,5); int clickX = random(714, 728); int clickY = random(233, 247); // log("src x: " + alchemyIcon.getAbsX() + " src: " + alchemyIcon.getAbsY()); mouse.click(clickX, clickY, false); // log("alch click x: " + clickX + " clickY: " + clickY); } public void clickHighSpellAlchemy() { // RS2Widget alchemyIcon = getWidgets().get(218, 21); // int clickX = alchemyIcon.getAbsX() + random(0,5); // int clickY = alchemyIcon.getAbsY() + random(0,5); int clickX = random(710, 730); int clickY = random(305, 320); // log("src x: " + alchemyIcon.getAbsX() + " src: " + alchemyIcon.getAbsY()); mouse.click(clickX, clickY, false); // log("alch click x: " + clickX + " clickY: " + clickY); } public void onPaint(Graphics2D g) { int mXp = getExperienceTracker().getGainedXP(Skill.MAGIC); super.onPaint(g); g.drawString("Magic XP: " + mXp, 387, 328); g.setColor(Color.CYAN); g.drawOval((int)mouse.getPosition().getX(), (int)mouse.getPosition().getY(), 10, 10); if(isInventoryOpen()) { g.drawRect(690, 210, 32, 32); } else { //g.drawRect(710, 230, 25, 25); g.drawRect(710, 300, 25, 25); } } private boolean isInventoryOpen() { RS2Widget inventoryWidget = getWidgets().get(149, 0, 3); return inventoryWidget != null && inventoryWidget.isVisible(); } }
- 1 reply
-
- 1
-
-
Thanks guys, glad you like it!
-
This script supports many fish such as shrimp, sharks, anglerfish etc. Start at canafis bank make sure you have the raw fish selected in your bank and it will make runs in between cooking them. package com.dexcooker; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import javax.swing.SwingUtilities; import org.osbot.rs07.api.map.Area; import org.osbot.rs07.api.model.RS2Object; import org.osbot.rs07.api.ui.RS2Widget; import org.osbot.rs07.api.ui.Tab; import org.osbot.rs07.script.Script; import org.osbot.rs07.script.ScriptManifest; import org.osbot.rs07.utility.ConditionalSleep; @ScriptManifest(author = "Dextrell", info = "Cooking Script", name = "DexCooker", version = 1.1, logo = "") public class DexCooker extends Script { private String selectedFish = null; private boolean guiComplete = false; private final Area BANK_AREA = new Area(2806, 3438, 2812, 3441); // Canifis Bank private final Area RANGE_AREA = new Area(2815, 3439, 2817, 3444); // Canifis Range @Override public void onStart() { // Create GUI to select fish SwingUtilities.invokeLater(this::createGUI); } /** * Creates the GUI for selecting the type of fish to cook. */ private void createGUI() { JFrame frame = new JFrame("DexCooker"); JPanel panel = new JPanel(); String[] fishOptions = { "Raw shrimps", "Raw sardine", "Raw herring", "Raw anchovies", "Raw trout", "Raw salmon", "Raw tuna", "Raw lobster", "Raw swordfish", "Raw monkfish", "Raw shark", "Raw anglerfish" }; JComboBox<String> fishSelection = new JComboBox<>(fishOptions); JButton startButton = new JButton("Start Cooking"); startButton.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { selectedFish = (String) fishSelection.getSelectedItem(); guiComplete = true; frame.dispose(); // Close the GUI after fish is selected } }); panel.add(new JLabel("Select fish to cook:")); panel.add(fishSelection); panel.add(startButton); frame.add(panel); frame.pack(); frame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE); frame.setLocationRelativeTo(null); // Center the frame frame.setVisible(true); } @Override public int onLoop() throws InterruptedException { if (!guiComplete) { return 100; // Wait until GUI is completed } // If the player is in the bank area and doesn't have fish to cook if (BANK_AREA.contains(myPlayer()) && !inventoryContainsRawFish()) { if (!getBank().isOpen()) { getBank().open(); if(getInventory().isFull()) getBank().depositAll(); sleep(1000); } else { if (getBank().contains(getRawFishID())) { getBank().withdrawAll(getRawFishID()); sleep(1000); } else { log("No more fish to cook. Stopping script."); stop(); } getBank().close(); } } // If player is at the range and has raw fish to cook if (RANGE_AREA.contains(myPlayer()) && inventoryContainsRawFish()) { if (!getTabs().isOpen(Tab.INVENTORY)) { getTabs().open(Tab.INVENTORY); sleep(500); } // Ensure the range is available RS2Object range = getObjects().closest("Range"); if (range != null) { if (!range.isVisible()) { getWalking().webWalk(RANGE_AREA); return 600; // Wait a bit after walking } // Interact with the range to start cooking if (range.interact("Cook")) { log("Interacting with the range to cook."); sleep(2000); // Wait for cooking animation to start RS2Widget cookingWidget = getWidgets().get(270, 14, 38); // Wait for the cooking widget to appear if (new ConditionalSleep(500, 1000) { @Override public boolean condition() throws InterruptedException { return cookingWidget != null && cookingWidget.isVisible(); } }.sleep()) { // Interact with the "Cook All" option in the widget if (cookingWidget != null) { // Widget 270, Child 14 is "Cook All" if(!cookingWidget.isVisible()) { range.interact("Cook"); } if (cookingWidget.interact("Cook")) { log("Selected 'Cook All' option."); // Wait until cooking starts (player animates) new ConditionalSleep(8000, 15000) { @Override public boolean condition() throws InterruptedException { return myPlayer().isAnimating(); } }.sleep(); } } else { log("Cook option not found."); } } // Wait until cooking is finished new ConditionalSleep(15000, 20000) { @Override public boolean condition() throws InterruptedException { return !myPlayer().isAnimating(); } }.sleep(); } } } // Walk to the bank if inventory is empty if (!inventoryContainsRawFish() && !BANK_AREA.contains(myPlayer())) { getWalking().webWalk(BANK_AREA); } // Walk to the range if the inventory contains raw fish if (inventoryContainsRawFish() && !RANGE_AREA.contains(myPlayer())) { getWalking().webWalk(RANGE_AREA); } return 300; // Delay for script loop } /** * Checks if the inventory contains the selected raw fish. * * @return true if inventory contains raw fish, false otherwise */ private boolean inventoryContainsRawFish() { return getInventory().contains(getRawFishID()); } /** * Maps the selected fish to its raw item ID. * * @return the raw fish item ID */ private int getRawFishID() { switch (selectedFish) { case "Raw shrimps": return 317; case "Raw sardine": return 327; case "Raw herring": return 345; case "Raw anchovies": return 321; case "Raw trout": return 335; case "Raw salmon": return 331; case "Raw tuna": return 359; case "Raw lobster": return 377; case "Raw swordfish": return 371; case "Raw monkfish": return 7944; case "Raw shark": return 383; case "Raw anglerfish": return 13439; default: return -1; } } @Override public void onExit() { log("Thank you for using DexCooker!"); } }
-
Clean and straightforward great work!
-
Try this
-
Now I'm curious, could you use Jython and have osbot as an export so you can use the OSBOT api on your python script, through a java class you can run jython and have a script manifest to start your script with the common methods used the python interpreter will execute the scripts functions through those methods? https://www.jython.org/ I'm not sure how this works under the hood but if it loads the files as an input stream that's inside the jar OSBot may throw some errors with that I've been having trouble loading anything as a resource...
-
Best web development framework to start with?
Dextrell replied to Qubit's topic in Software Development
Python Stack DJango + React Java Spring Boot + React For the database ask yourself if you need horizontal scaling if the answer is yes use NoSQL if you need relationships and ACID transactions over scaling horizontally use SQL -
Draynor Village Fisher/Banker [Open Source]
Dextrell replied to Lol_marcus's topic in Resource Collection
Looks good great job! Would you allow me to add on to this script? I'll give you credits of course -
I've tried loading the image as a resource countless of times i set a "res" folder as a resource my file structure is the following (I made sure the res folder was set as a resource folder) DexCutter -> src com.dexcutter <- code goes here -> res img.png It will always be null I've tried loading it many different ways even did DexCutter.class.getResourceAsStream The only thing I can consider doing is having a downloader that gets the image online and puts it in the data folder. try { backgroundImage = ImageIO.read(getScriptResourceAsStream("img.png")); } catch (IOException e) { log(e); }
-
Hello everyone this is my first script. I'd love to grab some feedback and any progress pictures you guys post would greatly help! This bot supports all trees around draynor village up to yew trees! Make sure to start the bot in lumbridge or draynor to get it running for all the other trees! If you select Magic Trees please be within the woodcutting guild! Source Code Media Jar Download