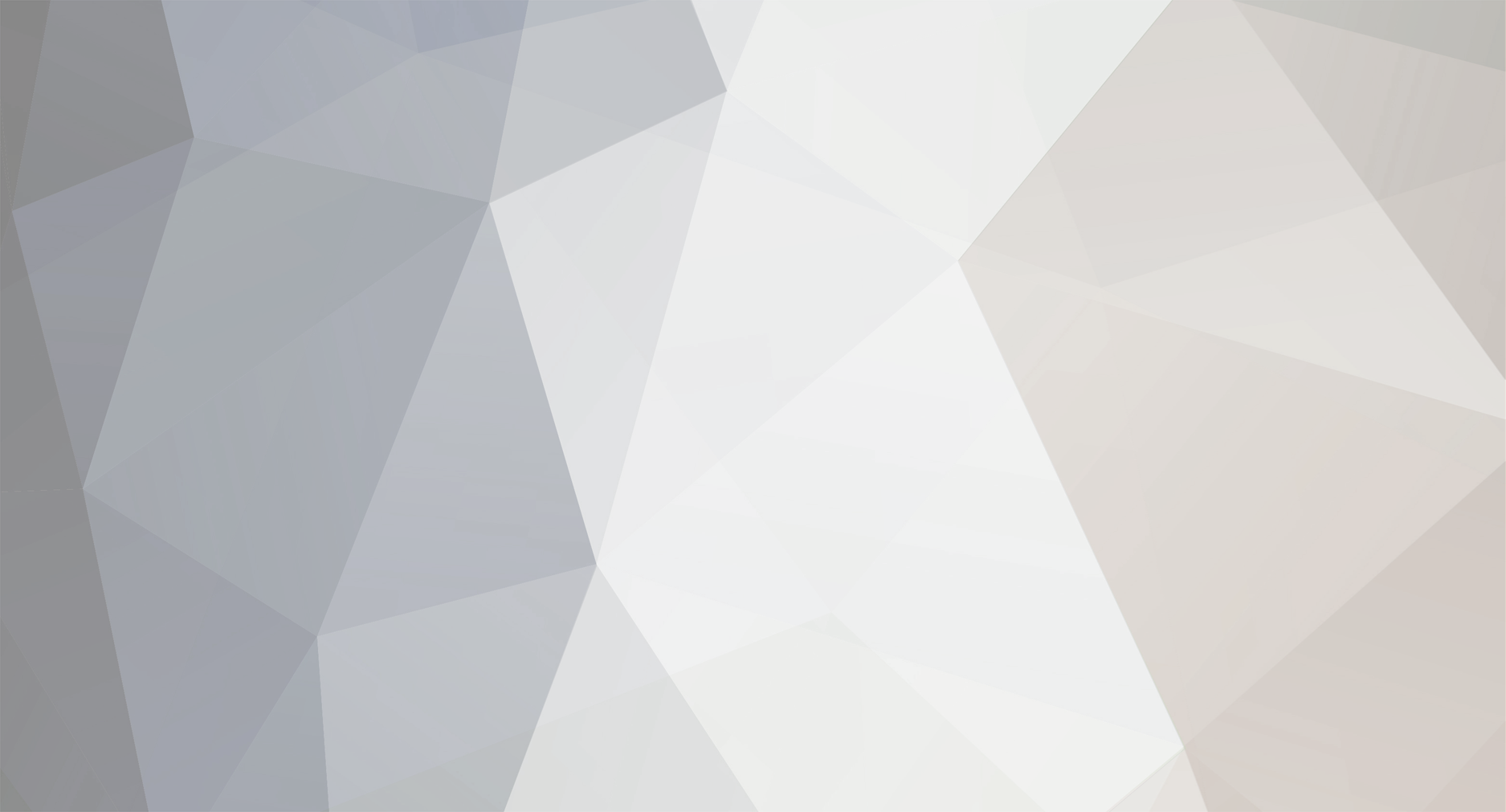
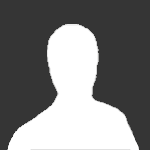
duhrealtim
Members-
Posts
6 -
Joined
-
Last visited
-
Days Won
1 -
Feedback
0%
duhrealtim last won the day on November 29 2023
duhrealtim had the most liked content!
Profile Information
-
Gender
Male
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
duhrealtim's Achievements

Newbie (1/10)
4
Reputation
-
key is a good location. no problems here so far
-
duhrealtim started following My first Woodcutting Script 1-15 and LF 82 Fishing accounts
-
LF 82 fishing accounts. If it has anglers its a plus. Doesn't have to.
-
Thank you!
-
I can send you a link to the new version of it. I recently changed it to willows and made it powerchop. Start it at draynor next to bank. WoodCutter.jar
-
import org.osbot.rs07.api.map.Area; import org.osbot.rs07.api.map.constants.Banks; import org.osbot.rs07.api.model.Entity; import org.osbot.rs07.api.model.Player; import org.osbot.rs07.api.model.RS2Object; import org.osbot.rs07.api.ui.Skill; import org.osbot.rs07.script.Script; import org.osbot.rs07.script.ScriptManifest; import org.osbot.rs07.utility.ConditionalSleep; @ScriptManifest(author = "Duhrealtim", name = "1-15 WoodCutter", info = "My First Script", version = 0.1, logo = "") public final class Woodcut extends Script { //Areas private final Area treeArea = new Area(3197, 3205, 3180, 3229); private final Area lumbridgeBankArea = new Area(3208, 3218, 3209, 3219); @Override public int onLoop() throws InterruptedException { //Keeps looping if (getInventory().getEmptySlotCount() != 0) { RS2Object tree = getObjects().closest(obj -> obj != null && obj.getName().equals("Tree") && getMap().canReach(obj)); //tree to chop if (!myPlayer().isAnimating()) { if (tree != null) { if (tree.interact("Chop down")) { new ConditionalSleep(5000, 2000) { @Override public boolean condition() throws InterruptedException { return false; } }.sleep(); } } } else if (tree == null) { // if it dont see tree it moves getCamera().toEntity(tree); log("Searching for a tree"); if (getWalking().webWalk(treeArea)) { log("Walking to trees"); new ConditionalSleep(5100, 6100) { @Override public boolean condition() throws InterruptedException { return false; } }; } } } else { log("Inventory Full."); //Walks to bank if (getWalking().webWalk(Banks.LUMBRIDGE_UPPER)) { //What bank it banks at. log("Walking to bank..."); new ConditionalSleep(5000, 1000) { @Override public boolean condition() throws InterruptedException { return true; } }; Entity depoBox = objects.closest("Bank deposit box"); //Opens deposit box and deposits logs. if (depoBox != null) { new ConditionalSleep(2000, 5000) { @Override public boolean condition() throws InterruptedException { return true; } }; if (depoBox.interact("Deposit")) { while (!depositBox.isOpen()) { new ConditionalSleep(2000, 5000) { @Override public boolean condition() throws InterruptedException { return true; } }; } depositBox.depositAllExcept("Bronze axe"); getWalking().webWalk(treeArea); } } } } return 1000; } } My very first script. Still trying to learn how to make it progressive.