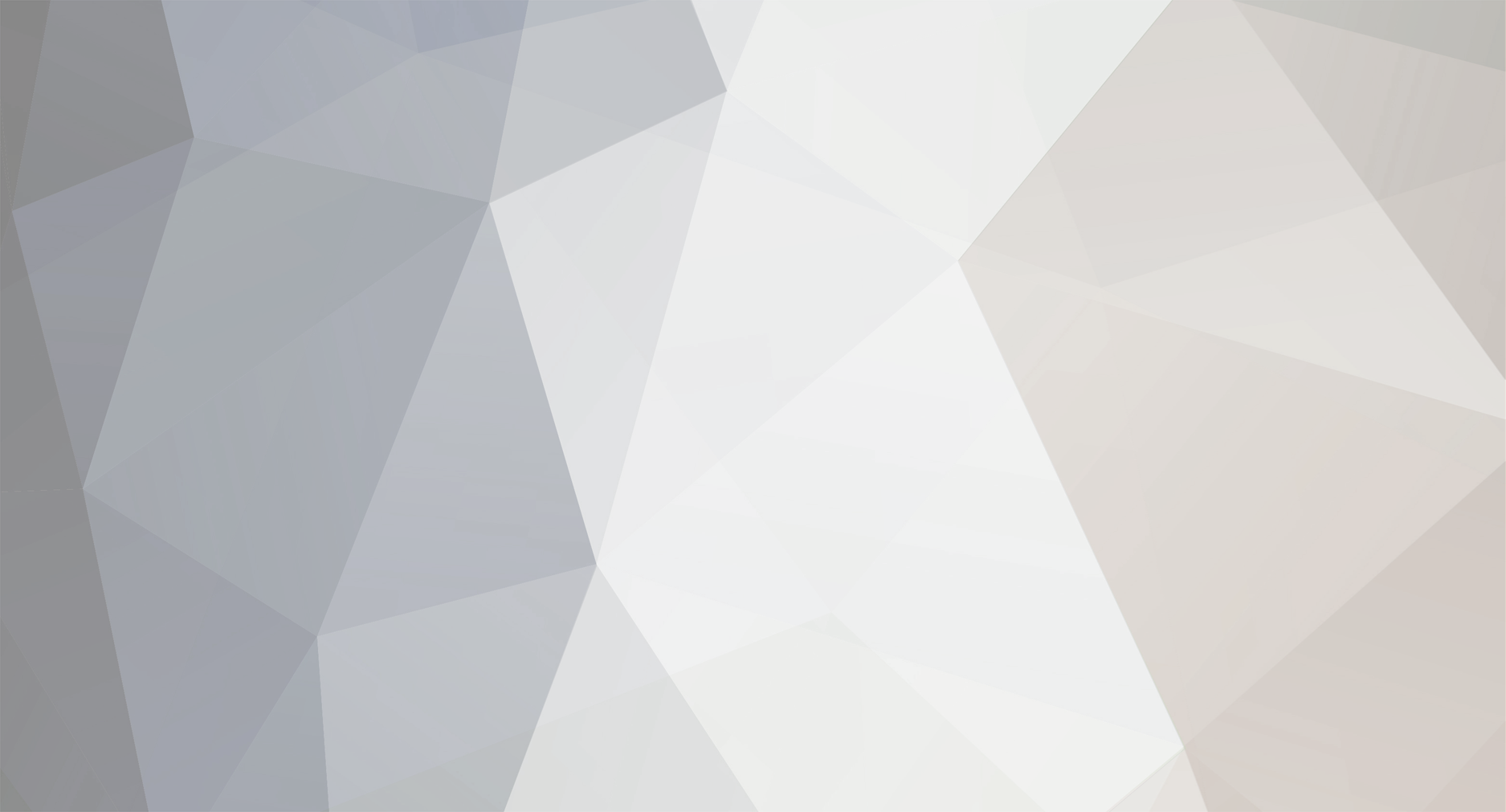
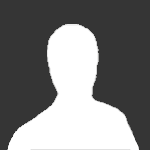
TiasJanos
Members-
Posts
3 -
Joined
-
Last visited
-
Feedback
0%
Profile Information
-
Gender
Male
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
TiasJanos's Achievements

Newbie (1/10)
0
Reputation
-
Nice code! I tried adapting it for gold amulets (u) and for Edgeville but for some reason when I change the banking areas and furnace area I break the code. At first it started continuously running back and forth and then I got it to bank but after withdrawing the bars it didn't go to the furnace. Any tips on how it can be adapted?
-
I also have issue with the bot getting stuck. It seems to happen mostly when the bot gets teleported back into the middle intersection of the mine.
-
Hi all! I was wondering if somebody could help me with my script. It chops trees alright but once the inventory is full it will not light them. import java.awt.Graphics2D; import org.osbot.rs07.script.Script; import org.osbot.rs07.script.ScriptManifest; import org.osbot.rs07.api.model.RS2Object; import org.osbot.rs07.utility.ConditionalSleep; import org.osbot.rs07.api.map.Area; @ScriptManifest(author = "Tias", info = "Cuts trees and burns logs", name = "Choppy and Burny", version = 0, logo = "") public class Main extends Script { @Override public void onStart() { log("Let's get started!"); } @Override public int onLoop() throws InterruptedException { int runEnergy = settings.getRunEnergy(); if (runEnergy > 50 && !settings.isRunning()) { settings.setRunning(true); } RS2Object tree = getObjects().closest(obj -> obj != null && obj.getName().equals("Tree") && getMap().canReach(obj)); //What tree to chop. if (!myPlayer().isAnimating() && !getInventory().isFull()) { if (tree != null) { if (tree.interact("Chop down")) { new ConditionalSleep(5000, 2000) { @Override public boolean condition() throws InterruptedException { return false; } }.sleep(); } } else if (tree == null) { //If it doesn't see a tree it moves the camera and then moves. Area treeArea = new Area(3143, 3239, 3198, 3205); getCamera().toEntity(tree); log("Searching for a tree..."); if (getWalking().webWalk(treeArea)) { log("Walking to trees..."); new ConditionalSleep(5000, 6000) { @Override public boolean condition() throws InterruptedException { return false; } }; } } else if (getInventory().isFull()) { log("Burning logs"); while (inventory.contains("Logs")) { log("looop"); if (inventory.isItemSelected()) { inventory.deselectItem(); } if(!(myPlayer().isAnimating()) && inventory.interact("Use", "Tinderbox")) { if (inventory.isItemSelected() && inventory.interactWithNameThatContains("Use", "Logs")) { new ConditionalSleep(3000) { @Override public boolean condition() { return (myPlayer().isAnimating()); } }.sleep(); } } } } } return random(200, 300); } @Override public void onExit() { log("Thanks for running!"); } @Override public void onPaint(Graphics2D g) { } }