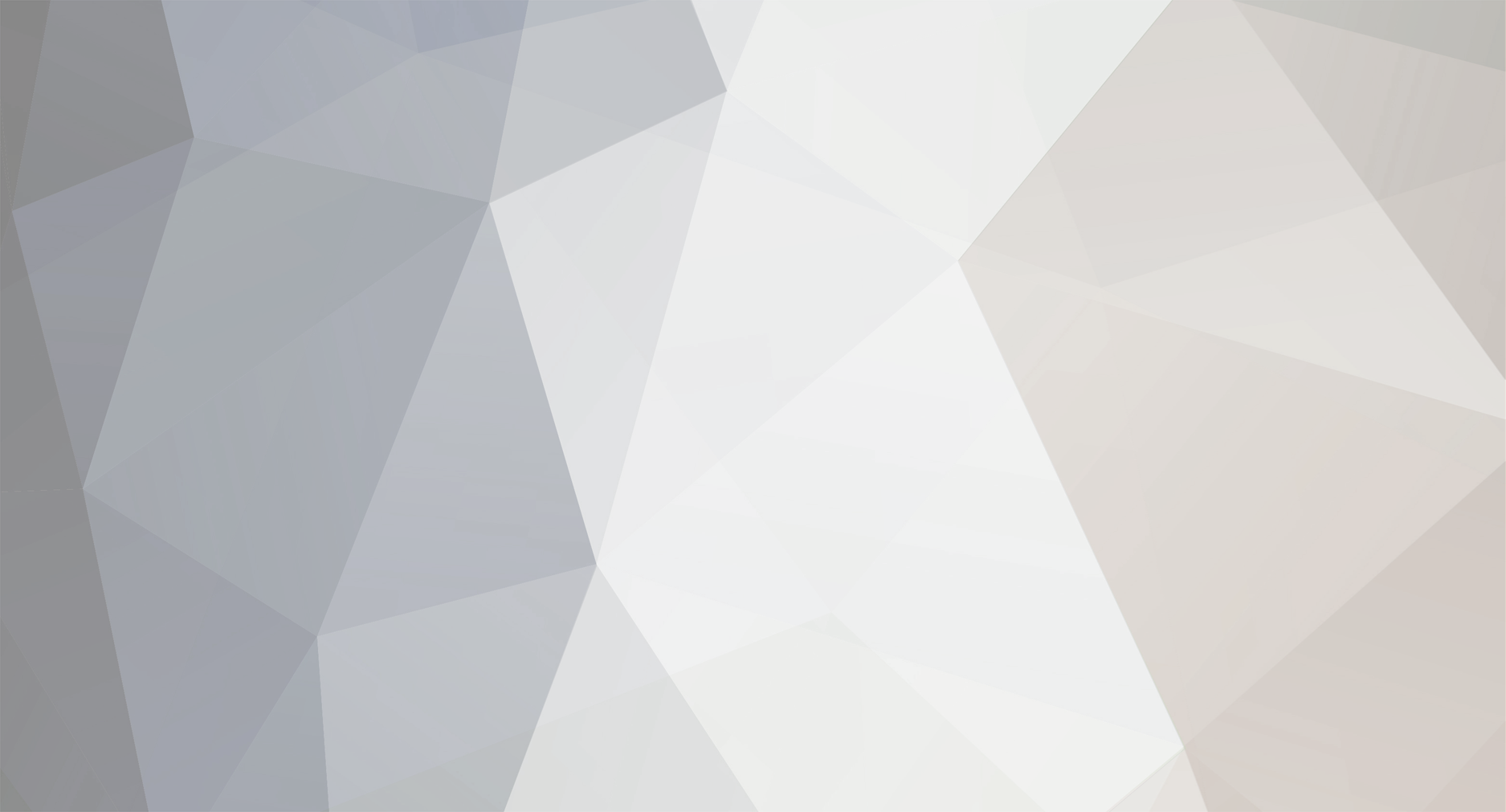
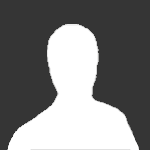
liverare
Scripter II-
Posts
1296 -
Joined
-
Last visited
-
Days Won
3 -
Feedback
0%
Everything posted by liverare
-
Pain spotted a missing class file. That is now resolved. Please download the new version and disregard the old! I've also cleaned up the packaging a bit.
-
Lol, you caught me there! I initially was going to return if ((on && i == 1) || (!on && i == 0)) as a bool itself rather than having the selector. This would have meant that returning false, whether the Setting menu wouldn't open or whether the Setting interface isn't valid, would have worked against the final conclusion: checking to see whether we interacted with the 'run' button and got the desired outcome. However, I strayed from that idea and decided to shove that condition in a selector and return true so I could implement a safer, conditional recursion. I decided to throw Runtime exceptions to allow developers to examine the source of the problem since, if you create a bool method that handles every problem by just returning false, there's no way to examine the source of the problem without debugging. <embarrassment> ...But I slacked on the new revision and, even though this is a bool method, at no point does it return false. </embarrassment> } else { j.getChild(34).interact(); boolean run = setRun(on); if (!run) throw new RuntimeException("Run toggling failed"); else return true; } to: } else { return j.getChild(34).interact() && setRun(on); /*setRun should return true from the first selector choice. If we didn't interact with the 'run' button, it'll return false before recurring the same method. } That will provide a false returnable, and I don't have to remove any other throwables.
-
Up yo' game, bitch ********a. Let's safe it like a motherfucker: /** * @param on * The state the run will be set to * @return * <tt>Run is toggled off/on to our desire</tt> * @throws InterruptedException * Interactions were interrupted */ public boolean setRun(boolean on) throws InterruptedException { // Find out the current state of "run" int i = client.getConfig(173); // Declare a new RS2Interface variable for later RS2Interface j = null; // Check to see if the state is on and that's our desired outcome, or // vice versa if ((on && i == 1) || (!on && i == 0)) return true; // Successful state--even if no interactions were // needed. Return true. // Check to see if we "can't" open Setting's tab else if (!openTab(Tab.SETTINGS)) { // We can't, so that's a problem. throw new RuntimeException("Unable to open the Setting's tab!"); // Define the interface variable and check to see if it doesn't // exist or it does, but isn't valid. } else if ((j = client.getInterface(261)) == null || !j.isValid()) { // The interface is either null or invalid, so that's a problem throw new RuntimeException( "We're in Settings, but the interface isn't valid!?"); /* * Final selector state, assume: * 1. Our desired Run state isn't selected * 2. We're in the Setting's tab * 3. The "j" RS2Interface variable exists and is valid */ } else { // Assume that there's no client change, so the child "34" is valid and interact. /* * EDITED CODE (thanks Xavier): * This function WILL ONLY RETURN FALSE if we did NOT interact * with the 'run' toggle-button. This condition will determine * whether another recursive check is exhibited. * * Recurse through this entire method again. The next recursion * should end right at the first selector that checks the desired * state to the actual state. * * Use the same parameter as before */ return j.getChild(34).interact() && setRun(on); } } Without comments, yo': /** * @param on * The state the run will be set to * @return * <tt>Run is toggled off/on to our desire</tt> * @throws InterruptedException * Interactions were interrupted */ public boolean setRun(boolean on) throws InterruptedException { int i = client.getConfig(173); RS2Interface j = null; if ((on && i == 1) || (!on && i == 0)) return true; else if (!openTab(Tab.SETTINGS)) { throw new RuntimeException("Unable to open the Setting's tab!"); } else if ((j = client.getInterface(261)) == null || !j.isValid()) { throw new RuntimeException( "We're in Settings, but the interface isn't valid!?"); } else { return j.getChild(34).interact() && setRun(on); } } Xavier caught a flaw in my first code-post, so I've updated them. The problem being: The old bool method wouldn't have returned false at any point, but rather; return true or throw exceptions. Good catch, Xavier. Hat's off to you, good sir.
-
getLoaded, getNearest, getSecondNearest, getFurthest and getRandom for RS2Objects, NPCs, Players and GroundItems. Enjoy: Download the class and source files here. Files hosted on Copy.com Open the Jar with any Zip/Rar program, and extract. There's pre-compiled classes (.class) and the source codes (.java). Enjoy. [edit] Forgot to mention: The available parameters are int, String and Filter Var-Args for every method! You don't have to have any parameter at all if you don't want. [/edit] Example of use: MethodAdapter methodAdapter = new MethodAdapter(this); final GroundItem bones = methodAdapter.getGroundItemHandler().getRandom( "Bones"); methodAdapter.interactWithEntity(bones, "Take", 1000, 200, 400, new XDynamicSleep() { @Override public boolean canAwake() { return !bones.exists(); } }); Or if you want to create an EntityXProfile: //Declare a new <code>MethodAdapter</code> instance final MethodAdapter m = new MethodAdapter(this); //Declare a new <code>EntityProfile<generic extends Entity> EntityXProfile<NPC> whiteKnight = new EntityXProfile<>(); //Provide the profile defining attributes: whiteKnight.setAreas(falador); whiteKnight.setNames("White Knight"); whiteKnight.setFilters(new Filter<NPC>() { @Override public boolean accept(NPC o) { return o.getFacing() == null || o.getFacing().equals(m.getLocalPlayer()); } }); //Check to make sure that the NPC isn't in combat or is in combat with me //Find the nearest and second nearest Rock Crab and declare new <code>NPC</code> instances NPC first = m.getNPCHandler().getNearest(whiteKnight); NPC second = m.getNPCHandler().getSecondNearest(whiteKnight); //Do what you want, just make sure you null-check them first. if (first != null && !first.isMoving()) first.interact("Attack"); else if (second != null && !first.isMoving()) second.interact("Attack"); else //No NPC found Description: I've finished creating a generic-orientated getter class for Entities. This ensures that objects, NPC, players and ground items all use the same methods to their specific needs. Because I'm a bit of a perfectionist, I prefer "getting" and evaluating object instances before interacting. I want to be the one who handles those processed. This helps ensure that each interaction is concluded flawlessly. How to install: Copy the src/co/ folder to your source folder.
-
/** * The <code>openTab(Setting)</code> will automatically check to make sure * that the desired tab is already present. If not, it will open it. * * Thereafter, we can check to see if we interact with the "run" toggle * button by returning whether we interacted with it via * <code>selectInterfaceOption(int, int)</code> * * @return * <tt>We interacted with the "run" toggle button</tt> * @throws InterruptedException * Interaction was interrupted */ public boolean toggleRun() throws InterruptedException { return openTab(Tab.SETTINGS) && selectInterfaceOption(261, 34); }
-
Depending on the distance between the points, you could have a mass cache of position instances that just aren't necessary. You should set dX and dY to an user-inputted "jump" offset. This would require additional coding to ensure the leaps don't overstep the end position, etc. The or operator in the while loop should be changed to an and operator, else the loop could prematurely stop before the entire path has been generated just because one axis has reached its max size. I think it would be more effective to work with a 2d array object instead of the ArrayList object. You can already compute the distance between those two points, so theoretically you can compute a fixed size amount--even with user-inputted offset parameter. This OCD implementation would prove more efficient (even if it's minuscule) than using an ArrayList. Then again, you can provide a capping value for the ArrayList instance. Why are you declaring int variables to represent the start and end X and Y positions? They're already get-able from the Position class. Just wasteful payload that doesn't need to exist. ..Just because: You don't need opening and closing brackets on selectors if they only execute one conditional operation. You could shave 2 lines off your code. I may update this post to ensure I haven't fooked up anywhere.
-
Rather than define new classes for each available NPC, create a new enum and implement your interface onto it and make the enum's constructor parameters accept the required info per profile. It'd be a nice way to document each task. Then again, the wide, vast variety of NPC and their combat preferences calls for a framework that's almost 100% adaptable, for instance; you have #getFinisher() method, but some tasks may require you to provoke the enemy NPC before combat can ensue.
-
Why? Your code is 74 lines long (2.34 KB) whereas mine is only 11 lines long (522 bytes). They both draw pretty much the same conclusion aside from mine lacking the walking handler. Program however you want, but condensing code whilst maintaining its overarching functionality and purpose is good practice. It helps remove redundant lines which help improve readability. [EDIT] I should also note that Pork has produced a much better method than both of ours because his uses some nice mathematics.
-
When even bother scrolling? This is a re-post of my submitted code on the Withdraw without scrolling bug thread: public static RectangleDestination getScrollerDes(Script script, int id) { if (!script.client.getBank().isOpen() || !script.client.getBank().contains(id)) return null; int i = 0; for (Item next : script.client.getBank().getItems()) if (next != null && next.getId() == id) break; else i++; i /= 2; return new RectangleDestination(new Rectangle(480, 68 + i, 15, 4)); }
-
Perhaps you should simplify your code to something like: public static Position getNextPosition(Script script, Position end) { Position cur = script.myPosition(); int disX = end.getX() - cur.getX(), disY = end.getY() - cur.getY(); boolean revX = disX < 0, revY = disY < 0; disX = Math.abs(disX); disY = Math.abs(disY); if (disX >= 17) disX = 10 + MethodProvider.random(5); if (disY >= 17) disY = 10 + MethodProvider.random(5); return new Position(cur.getX() + (revX ? ~disX : disX), cur.getY() + (revY ? ~disY : disY), cur.getZ()); } I made my method a 'getter' to allow users to provide users with the ability to handle the actual walking themselves.
-
I think this could help (night-time 'throw-together'): public static RectangleDestination getScrollerDes(Script script, int id) { if (!script.client.getBank().isOpen() || !script.client.getBank().contains(id)) return null; int i = 0; for (Item next : script.client.getBank().getItems()) if (next != null && next.getId() == id) break; else i++; i /= 2; return new RectangleDestination(new Rectangle(480, 68 + i, 15, 4)); }
-
If I were to create an antiban--which I wouldn't because, unless they provide robust in-game interactions, they're redundant and 100% placebo--I would implement interactions "antibans" in an abstract enum and call for a random valid index, and probably allow for a queue of indices to be iterated through.
-
...Removing factual criticism. Sad. I'll post it again in short-form: If you've read the source for CowHideKiller or XYewCutter, you will know that those bolded keywords aren't reflected in either of his scripts. Dude don't post here... not going to say this again. Honestly neither of those scripts are a result of the project or are what that statement is directed towards. I suppose I am being unnecessarily nit-picky. I'm sure a collaboration will yield good quality scripts, but it all falls down to the members of that group, and if you're planning to lead this group, unless you've got a fuck-of-a-good programmer, I doubt there would be much of an improvement--which would go against your bold statement. Good luck on your future projects!
-
...Removing factual criticism. Sad. I'll post it again in short-form: If you've read the source for CowHideKiller or XYewCutter, you will know that those bolded keywords aren't reflected in either of his scripts.
-
For dynamic sleeping: 1. Create a new interface XDynamicSleep with an abstract canAwake method. public interface XDynamicSleep { public boolean canAwake(); } 2. If you've got a script framework, implement this into it, else just throw it in your script somewhere: public boolean sleep(long base, long offset, XDynamicSleep sleep) throws InterruptedException { if (base < 0 || sleep == null) throw new IllegalArgumentException(); long start = System.currentTimeMillis(); base += MethodProvider((int) offset); offset = (long) (base * 0.1); while (start + base > System.currentTimeMillis()) { if (sleep.canAwake()) return true; else sleep(offset); } return false; } 3. Time to sleep! Example: . . . if (goblin.interact("attack")) if (sleep(3000, 2000, new XDynamicSleep() { @Override public boolean canAwake() { return !goblin.exists(); } }) ) { //Sleep was interrupted, goblin must have died } else { //Sleep slept to the full length... Time to revalidate //our situation! } . . . I wrote this up in NotePad++ so there might be syntax errors.
-
What about generating dynamic nodes upon game entry? Is that included, too?
-
Are you going to take advantage of settings?
-
This is helpful! I didn't realize client#getCurrentRegion()#getObjects() existed. Now I have my own ObjectX class that has convenient functions–like yours, except mine's tailored more towards my needs! So, thanks for that!
-
I've rewritten my code to search even if a search result is already present. Though, this one is more organized and uses resources outside of the class itself.
-
Wrote this up right quick: import org.osbot.script.MethodProvider; import org.osbot.script.Script; import org.osbot.script.rs2.ui.Bank; import org.osbot.script.rs2.ui.EquipmentSlot; import org.osbot.script.rs2.ui.RS2Interface; import org.osbot.script.rs2.ui.RS2InterfaceChild; //#click() is depricated, if it doesn't work, replace for #interact(...) public class BankSearch extends Bank { public static final int BANK_PARENT = 12; public static final int BANK_CHILD = 0; public static final int BANK_CHILD_TITLE = 2; public static final int BANK_CHILD_SEARCH = 20; public static final int BANK_CHILD_DEP_INV = 22; public static final int BANK_CHILD_DEP_ARM = 24; public static final int BANK_SEARCH_PARENT = 548; public static final int BANK_SEARCH_CHILD = 94; //public static final String BANK_TITLE = "The Bank of RuneScape"; //public static final String BANK_TITLE_SEARCH = "Showing items: <col=ff0000></col>"; private final Script script; public BankSearch(Script script) { super(script.bot, script.client); this.script = script; } @Override public boolean isOpen() { return getWidgetChild(BANK_PARENT, BANK_CHILD) != null; } @SuppressWarnings("deprecation") public boolean depositInventory() throws InterruptedException { RS2InterfaceChild i = getWidgetChild(BANK_PARENT, BANK_CHILD_DEP_INV); return i != null && i.click() && script.client.getInventory().isEmpty(); } @SuppressWarnings("deprecation") public boolean depositEquipment() throws InterruptedException { RS2InterfaceChild i = getWidgetChild(BANK_PARENT, BANK_CHILD_DEP_ARM); return i != null && i.click() && isNaked(); } @SuppressWarnings("deprecation") public boolean enterSearchMode() throws InterruptedException { RS2InterfaceChild i = getWidgetChild(BANK_PARENT, BANK_CHILD_SEARCH); try { return i != null && i.click() && isInSearchMode(); } finally { sleep(1000 + MethodProvider.random(1000), new DynamicSleep() { @Override public boolean canAwake() { return isInSearchMode(); } }); } } @Deprecated //Poor fucking coding...tired. public boolean invokeSearch(String string) throws InterruptedException { if (!isInSearchMode() || !isSearchEngineOpen()) return false; String cur = getCurrentlyEnteredSearchText(); if (cur.equalsIgnoreCase(string)) return true; else if (isSearchEngineOpen() || enterSearchMode()) script.type(string); return (cur = getCurrentlyEnteredSearchText()).equalsIgnoreCase(string); } public String getCurrentlyEnteredSearchText() { RS2InterfaceChild i = getWidgetChild(BANK_SEARCH_PARENT, BANK_SEARCH_CHILD); if (i == null) return null; String s = i.getMessage(); return s.substring(0, s.length() - 1); // -1 for * } public boolean isSearchEngineOpen() { RS2InterfaceChild i = getWidgetChild(BANK_SEARCH_PARENT, BANK_SEARCH_CHILD); return i != null && i.isVisible(); } public boolean isInSearchMode() { RS2InterfaceChild i = getWidgetChild(BANK_PARENT, BANK_CHILD_TITLE); return i != null && i.getMessage().startsWith("Showing"); } public String getSearchedPhrase() { RS2InterfaceChild i = getWidgetChild(BANK_PARENT, BANK_CHILD_TITLE); if (!isInSearchMode()) return null; String s = i.getMessage(); return s.length() - 6 != 27 ? s.substring(27, s.length() - 6) : ""; } private boolean isNaked() { for (EquipmentSlot next : EquipmentSlot.values()) if (script.equipmentTab.getItemInSlot(next) != null) return false; return true; } private RS2InterfaceChild getWidgetChild(int a, int b) { RS2Interface p = script.client.getInterface(a); return p != null && p.isValid() ? p.getChild(b) : null; } private synchronized void sleep(long timeout, DynamicSleep condition) throws InterruptedException { final long old = System.currentTimeMillis(); while (old + timeout >= System.currentTimeMillis()) if (condition.canAwake()) break; else script.sleep(50 + MethodProvider.random(200)); } static interface DynamicSleep { boolean canAwake(); } } Example of it in use: import javax.swing.JOptionPane; import org.osbot.script.Script; import org.osbot.script.ScriptManifest; import co.uk.liverare.framework.wrapper2.BankSearch; @ScriptManifest(name = "Demo", info = "Script designed for testing purposes.", version = 1.0, author = "LiveRare") public class Demo extends Script { BankSearch bankSearch; String search; @Override public void onStart() { bankSearch = new BankSearch(this); search = JOptionPane.showInputDialog("Search:"); } @Override public int onLoop() throws InterruptedException { if (bankSearch.isOpen()) { if (bankSearch.isSearchEngineOpen()) bankSearch.invokeSearch(search); sleep(2000 + random(1000)); } return 100; } } #isOpen() overriden to work #depositInventory() doesn't check if inventory is empty first--you check for that #depositEquipment() doesn't check if naked first--you do that #enterSearchMode() enters the search mode #invokeSearch(String string) enters text into the search engine #getCurrentlyEnteredSearchText() gets the text in the search engine #isSearchEngineOpen() <tt>search engine exists, and is valid and visible</tt> #isInSearchMode() <tt>bank is in "search mode"</tt> #getSearchedPhrase() returns the searched phrase located in the bank's title
-
Why are you running 2 separate selectors? if(currentTab() == Tab.INVENTORY) { ... } if(currentTab() == Tab.EQUIPMENT) { ... } Only one tab can be opened at any given time, so use else if for the 2nd selector, else if the first selector is true (inventory), you will also be redundantly checking to see if the equipment tab is open as well. Also, the fuck is this?: if(currentTab() == Tab.INVENTORY) { ... if(currentTab() != Tab.INVENTORY) return; ... } if(currentTab() == Tab.EQUIPMENT) { ... if(currentTab() != Tab.EQUIPMENT) return; ... } You don't need to check to see if we're not in the inventory tab once we've already checked to see if we're in the damn inventory tab. Same for the equipment tab.
-
Shameless bump, yo'.
-
And who is he? I'll see if he's up for a collaboration. Oh, his name is actually Dashboard, lol.
-
Correct! To make this an actual bot would be...extensive. And, as for the guy making a fully automated Clue' bot, well... I doubt it'll support all 3 levels. Maybe the first level of clue scrolls because I've seen that done before. But the 2nd and 3rd? That's a challenge. I'm not saying making a fully automated bot is impossible, but it'd require a fully functioning, fail-safe'd Web Walker & teleporter. It'd also require a ton of environment fail-safes because clues can lead you into the wilderness, and there's no way to (as of yet) detect players' combat levels to determine if they're a threat.