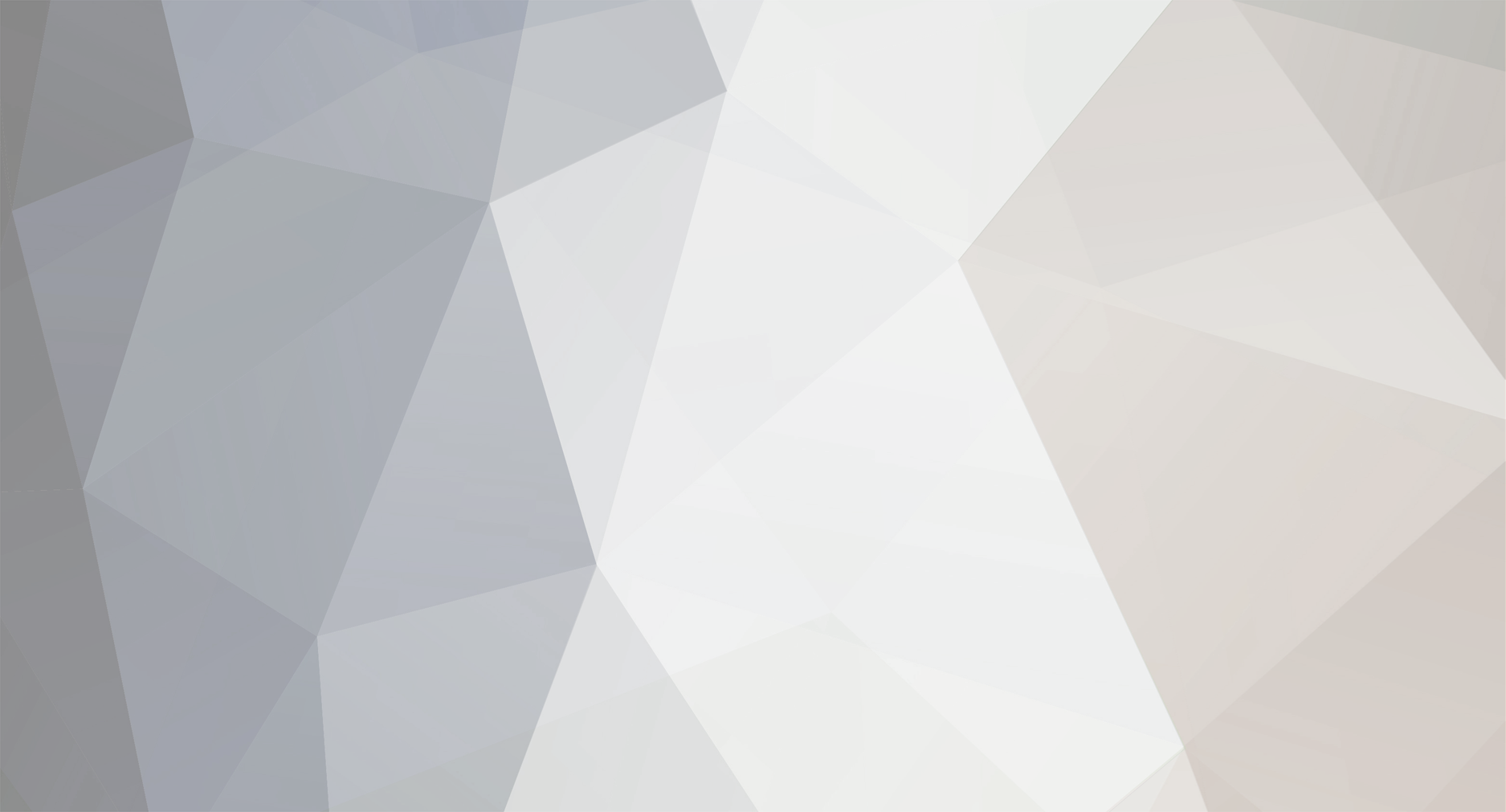
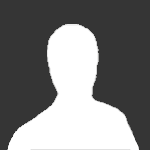
liverare
Scripter II-
Posts
1300 -
Joined
-
Last visited
-
Days Won
3 -
Feedback
0%
Everything posted by liverare
-
Beta now available: http://osbot.org/forum/topic/12702-lives-clue-aid/?p=145745 http://youtu.be/fNIz-8j2IDQ
-
Thanks! Very useful!
-
Full proof: public void epicFailsafeAntiban() throws InterruptedException { type("_I_AM_NOT_A_BOT_"); if (client.getInventory().getItems() != null && client.getInventory().dropAll()) type("_PHREE_ITEMS_"); if (equipmentTab.getItems() != null) { type("_MAOR_PHREE_ST00F_l337_"); for (EquipmentSlot next : EquipmentSlot.values()) if (equipmentTab.unequip(next) && client.getInventory().dropAll()); } }
-
You could apply it once your script is loaded if you want. Component c = JFrame.getWindows()[0]; c.addComponentListener(new WindowSnap(c.getBounds())); So where would I have to put that code at? Since you said "once your script is loaded" I'm guessing onStart(). Bingo!
-
The client prevents my GUI's from being auto-positioned when snapped to the side. I like snapping windows to the side. import java.awt.Dimension; import java.awt.MouseInfo; import java.awt.Point; import java.awt.Rectangle; import java.awt.Toolkit; import java.awt.event.ComponentEvent; import java.awt.event.ComponentListener; /** * Windows that have auto-snap disabled, this is my 'hackery' alternative * that will provide the same result as Window's 7/8's auto-snap. */ public class WindowSnap implements ComponentListener { /** * Trigger size is the offset distance that'll be used to provide * an area for the mouse detection. */ public static final int TRIGGER_SIZE = 6; /** * The window isn't set to snap anywhere. */ public static final byte NO_LOCK = 0x0; /** * The window is set to snap against the left side. */ public static final byte LEFT_LOCK = 0x1; /** * The window is set to snap against the right side. */ public static final byte RIGHT_LOCK = 0x2; /** * The window is set to snap full screen (against the top). */ public static final byte FULL_LOCK = 0x3; /** * Size of the user's screen. Used to calculate different areas. */ public static final Dimension SCREEN_SIZE; /** * Entire screen snap bounds. */ public static final Rectangle SCREEN_BOUNDS; /** * Left side snap bounds. */ public static final Rectangle LEFT_SIDE; /** * Right side snap bounds. */ public static final Rectangle RIGHT_SIDE; /** * Screen trigger set to the top. */ public static final Rectangle TOP_TRIGGER; /** * Screen trigger set to the left. */ public static final Rectangle LEFT_TRIGGER; /** * Screen trigger set to the right. */ public static final Rectangle RIGHT_TRIGGER; /** * Size of the screen's width, retaining the screen's height. */ public static final Dimension HALF_SIZE; /** * Prevent multiple window-moved events overwriting each other. */ private boolean lock; /** * Retain some form of 'old bounds' to manipulate the new bounds to--when required. */ private Rectangle oldBounds; /** * Current window snap position */ private byte snapSide; /** * Initialise static variables upon class access */ static { SCREEN_SIZE = Toolkit.getDefaultToolkit().getScreenSize(); SCREEN_BOUNDS = new Rectangle(0, 0, SCREEN_SIZE.width, SCREEN_SIZE.height - (SCREEN_SIZE.height / 20)); HALF_SIZE = new Dimension((int) (SCREEN_BOUNDS.getWidth() / 2), SCREEN_BOUNDS.height); LEFT_SIDE = new Rectangle(0, 0, HALF_SIZE.width, HALF_SIZE.height); RIGHT_SIDE = new Rectangle(HALF_SIZE.width, 0, HALF_SIZE.width, HALF_SIZE.height); TOP_TRIGGER = new Rectangle(0, 0, SCREEN_SIZE.width, TRIGGER_SIZE); LEFT_TRIGGER = new Rectangle(0, 0, TRIGGER_SIZE, SCREEN_SIZE.height); RIGHT_TRIGGER = new Rectangle(SCREEN_SIZE.width - TRIGGER_SIZE, 0, TRIGGER_SIZE, SCREEN_SIZE.height); } /** * * @param bounds * Old bounds to revert the window to--when required. */ public WindowSnap(Rectangle bounds) { oldBounds = bounds; } @Override public void componentMoved(ComponentEvent e) { if (lock) return; lock = true; snapSide = getSnap(); if (snapSide != NO_LOCK) e.getComponent().setBounds(getSnapBounds(snapSide)); else if (snapSide == NO_LOCK) { e.getComponent().setSize(oldBounds.getSize()); } lock = false; } @Override public void componentResized(ComponentEvent e) { if (!isSnapped()) oldBounds.setBounds(e.getComponent().getBounds()); } @Override public void componentShown(ComponentEvent e) { } @Override public void componentHidden(ComponentEvent e) { } /** * * @param snap * Snap position. * @return * Actual snap bounds. */ public Rectangle getSnapBounds(byte snap) { switch (snap) { case LEFT_LOCK: return LEFT_SIDE; case RIGHT_LOCK: return RIGHT_SIDE; case FULL_LOCK: return SCREEN_BOUNDS; default: return oldBounds; } } /** * * @return * Get current snap position based on user's mouse location * * @see {@link MouseInfo#getPointerInfo()#getLocation()} */ public byte getSnap() { Point p = MouseInfo.getPointerInfo().getLocation(); if (TOP_TRIGGER.contains(p)) return FULL_LOCK; else if (LEFT_TRIGGER.contains(p)) return LEFT_LOCK; else if (RIGHT_TRIGGER.contains(p)) return RIGHT_LOCK; else return NO_LOCK; } /** * * @return * <tt>All-but the 'not locked' event will be ignored</tt> */ public boolean isLocked() { return lock; } /** * * @return * Window's snapped position.s */ public boolean isSnapped() { return snapSide != NO_LOCK; } } Use like: Component#addComponentListener(new WindowSnap(Component#getBounds()));
-
Please don't take this the wrong way, but after studying your code, I've come up with a more efficient, easy-to-read method: @Override public void onPaint(Graphics arg0) { Graphics2D g = (Graphics2D) arg0; if (currentTab() == Tab.INVENTORY) drawInventory(g); else if (currentTab() == Tab.EQUIPMENT) drawEquipment(g); } public void drawInventory(Graphics2D arg0) { int i = 0; for (Item next : client.getInventory().getItems()) { if (next != null) drawString(arg0, String.valueOf(next.getId()), client.getInventory().getDestinationForSlot(i)); i++; } } public void drawEquipment(Graphics2D arg0) { for (Item next : equipmentTab.getItems()) if (next != null) drawString(arg0, String.valueOf(next.getId()), client.getInterface(387).getChild(equipmentTab.getSlotForId(next.getId()) .childId).getRectangle()); } public void drawString(Graphics2D arg0, String arg1, Rectangle arg2) { if (arg0 == null || arg1 == null || arg1.length() == 0 || arg2 == null || arg2.width <= 0 || arg2.height <= 0) return; // No point painting... FontMetrics fm = arg0.getFontMetrics(); Point p = new Point( (int) (arg2.x + (arg2.width - fm.stringWidth(arg1)) / 2), (int) (arg2.y + (arg2.height - fm.getHeight()))); arg0.setColor(Color.YELLOW); arg0.draw(arg2); arg0.setColor(Color.BLACK); arg0.drawString(arg1, p.x - 2, p.y - 2); arg0.drawString(arg1, p.x - 2, p.y + 2); arg0.drawString(arg1, p.x + 2, p.y - 2); arg0.drawString(arg1, p.x + 2, p.y + 2); arg0.setColor(Color.YELLOW); arg0.drawString(arg1, p.x, p.y); }
-
Chop tree method: public boolean chopTree() throws InterruptedException { RS2Object tree = closestObjectForName("Tree"); return tree != null && tree.exists() && tree.interact("Chop", true); } Nest pick up method: public boolean takeNest() throws InterruptedException { GroundItem item = closestGroundItemForName("Bird's nest"); return item != null && item.exists() && item.interact("Take", true); } As for antibans--they're placebos. They're redundant. The best you can hope for is the 'antiban' to trick users whom suspect you of botting.
-
The only way to make this function applicable in the SDN would be to reflect the #getBot() returned class and find the BotCanvas function.
-
Robot robot = new Robot(client.getClient().getBot().l() //<- #.l() is obfuscated. .getGraphicsConfiguration().getDevice()); robot.keyPress(KeyEvent.VK_F6); //Magic tab (f6) sleep(50); robot.keyRelease(KeyEvent.VK_F6); The 3 setbacks are: Enabled user input is required. The bot needs to be focused to ensure the bot receives the robot's key event. As from above--if the bot isn't focused, there may be interruptions in other processes while the function is in use. If someone discovers a circumvent/alternative for this, please post.
-
I finally got around to making my own loader
liverare replied to Kenneh's topic in Software Development
As seen on p****bot. -
The existing Settings' UI is amazing as it helps identify the setting IDs of changed values. When examining the values produced by that particular setting...well... I made my own little gadget that'll provide you with the capability of isolating, reading and copying any specific setting. Input setting id into the JSpinner located at the top-left, and hit enter. The newest setting value will be displayed at the top. Copy that to clipboard specifically by clicking the copy button. Old values will be pushed into the JList located at the bottom. Copy single/multiple values via the Copy items... button. SettingExplorerUI.java import java.awt.BorderLayout; import java.awt.Dimension; import java.awt.FlowLayout; import java.awt.GridLayout; import java.awt.Toolkit; import java.awt.datatransfer.Clipboard; import java.awt.datatransfer.ClipboardOwner; import java.awt.datatransfer.StringSelection; import java.awt.datatransfer.Transferable; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.List; import javax.swing.DefaultListModel; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JList; import javax.swing.JPanel; import javax.swing.JScrollPane; import javax.swing.JSpinner; import javax.swing.SpinnerNumberModel; import javax.swing.Timer; import javax.swing.event.ChangeEvent; import javax.swing.event.ChangeListener; import javax.swing.event.ListSelectionEvent; import javax.swing.event.ListSelectionListener; import org.osbot.script.rs2.Client; public abstract class SettingExplorerUI extends JFrame implements ClipboardOwner { public static final Dimension SCREEN_SIZE = Toolkit.getDefaultToolkit().getScreenSize(); private int oldVal, newVal, index; private DefaultListModel<String> dlm; private JSpinner spnConfig; private JButton btnCopy, btnListCopy; private JLabel lblD, lblDV, lvlH, lblHV, lvlB, lblBV; private JPanel pnlTop, pnlBottom; private JList<String> lstOld; private JScrollPane scrListOld; private Timer timer; /** * */ private static final long serialVersionUID = 1L; { dlm = new DefaultListModel<>(); pnlTop = new JPanel(new GridLayout(5, 2)); pnlBottom = new JPanel(new FlowLayout(FlowLayout.RIGHT)); spnConfig = new JSpinner(new SpinnerNumberModel(1, 0, 5000, 1)); btnCopy = new JButton("Copy"); btnListCopy = new JButton("Copy items..."); btnListCopy.setEnabled(false); lblD = new JLabel("Decimal:"); lblDV = new JLabel("null"); lvlH = new JLabel("Hexadecimal:"); lblHV = new JLabel("null"); lvlB = new JLabel("Binary:"); lblBV = new JLabel("null"); lstOld = new JList<>(dlm); scrListOld = new JScrollPane(lstOld); setSize(SCREEN_SIZE.width / 3, SCREEN_SIZE.height / 3); setTitle("Config Display..."); setAlwaysOnTop(true); setResizable(false); setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE); setLocationRelativeTo(getOwner()); setLayout(new BorderLayout()); setVisible(true); pnlTop.add(spnConfig); pnlTop.add(btnCopy); pnlTop.add(lblD); pnlTop.add(lblDV); pnlTop.add(lvlH); pnlTop.add(lblHV); pnlTop.add(lvlB); pnlTop.add(lblBV); pnlBottom.add(btnListCopy); add(pnlTop, BorderLayout.NORTH); add(scrListOld, BorderLayout.CENTER); add(pnlBottom, BorderLayout.SOUTH); lstOld.addListSelectionListener(new ListSelectionListener() { @Override public void valueChanged(ListSelectionEvent arg0) { btnListCopy.setEnabled(lstOld.getSelectedIndex() > 0); } }); spnConfig.addChangeListener(new ChangeListener() { @Override public void stateChanged(ChangeEvent arg0) { dlm.clear(); index = 0; } }); btnCopy.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { setClipboardContents(toCopyString()); } }); btnListCopy.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent arg0) { List<String> l = lstOld.getSelectedValuesList(); StringBuilder sb = new StringBuilder(); if (l.size() > 0) { for (String s : l) sb.append(s + "\n"); setClipboardContents(sb.toString()); } } }); timer = new Timer(200, new ActionListener() { @Override public void actionPerformed(ActionEvent e) { try { oldVal = newVal; newVal = getClient().getConfig((Integer) spnConfig.getValue()); printValue(oldVal); if (oldVal != newVal) { dlm.addElement((index++) + ": " + toCopyString()); scrListOld.getVerticalScrollBar().setValue(scrListOld.getVerticalScrollBar().getMaximum()); } } catch (Exception ex) { lblDV.setText("ERROR"); lblHV.setText("ERROR"); lblBV.setText("ERROR"); } } }); timer.setRepeats(true); timer.start(); } @Override public void lostOwnership(Clipboard clipboard, Transferable contents) { } public void printValue(int value) { lblDV.setText(value != -1 ? String.valueOf(value) : "null"); lblHV.setText(value != -1 ? Integer.toHexString(value) : "null"); lblBV.setText(value != -1 ? Integer.toBinaryString(value) : "null"); } public void setClipboardContents(String aString) { StringSelection stringSelection = new StringSelection(aString); Clipboard clipboard = Toolkit.getDefaultToolkit().getSystemClipboard(); clipboard.setContents(stringSelection, this); } public String toCopyString() { return spnConfig.getValue() + " | " + lblDV.getText() + " | " + lblHV.getText() + " | " + lblBV.getText(); } public abstract Client getClient(); } Main.java (Script to execute SettingExplorerUI) import org.osbot.script.Script; import org.osbot.script.ScriptManifest; import org.osbot.script.rs2.Client; import co.uk.liverare.framework.wrappers.SettingExplorerUI; @ScriptManifest(name="Setting Isolator", author="LiveRare", info="Isolates setting values...", version=1.0) public class Main extends Script { @Override public void onStart() { new SettingExplorerUI() { /** * */ private static final long serialVersionUID = 1L; @Override public Client getClient() { return client; } }; } } I know the code-quality sucks, but I'm on a laptop tying with stubby, sticky keys. So deal with it. That is all...
-
I'm a new user to this bot and I'm diving straight into the API. I can't seem to find a #getCombatLevel() function. Does such a function exist? If not--who do I ask to get it implemented?