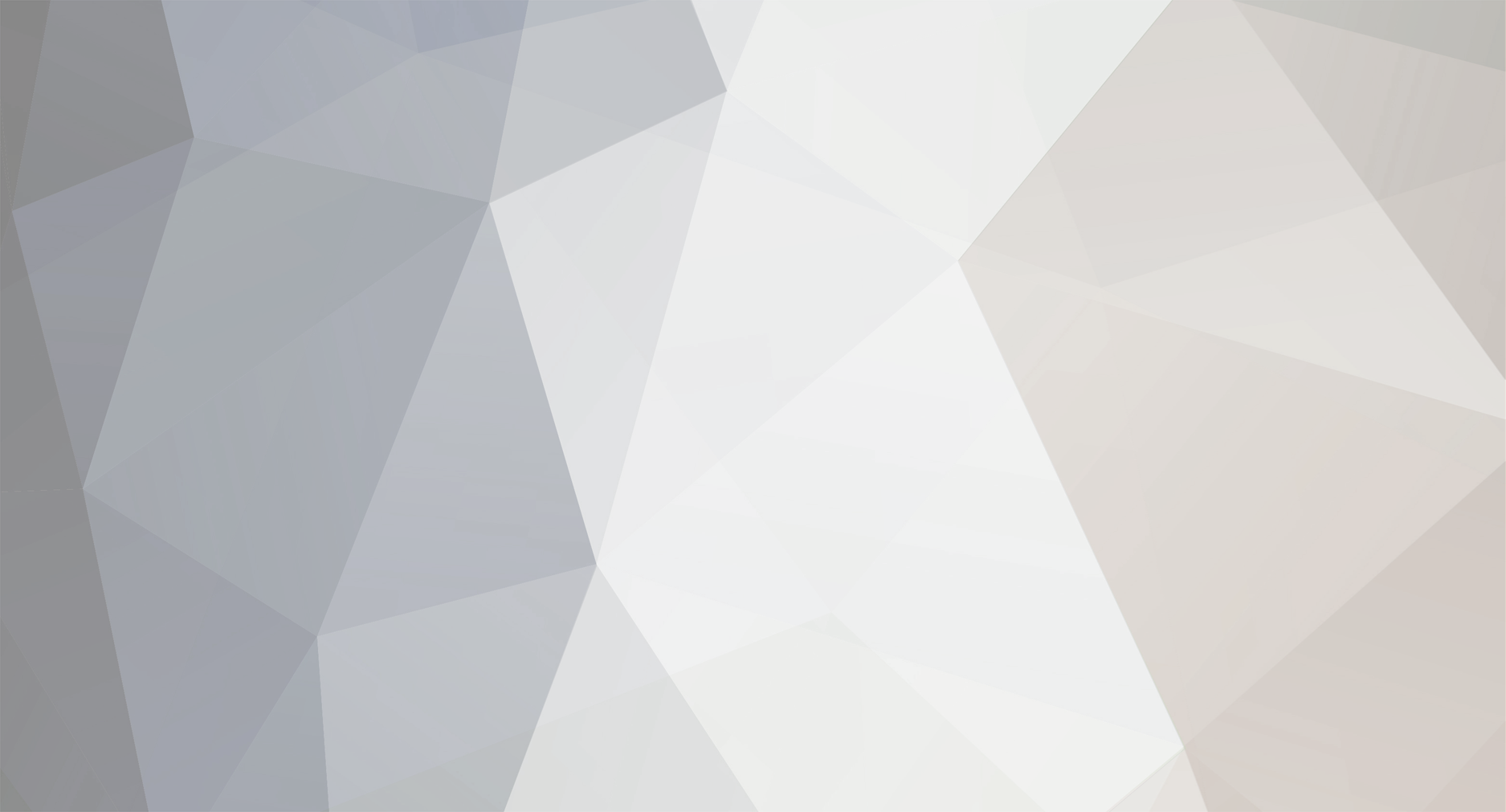
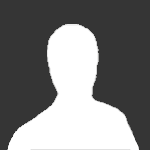
killsmh
-
Posts
63 -
Joined
-
Last visited
-
Feedback
100%
Posts posted by killsmh
-
-
getBot().addMessageListener(f2pWc.this);
@Override
public void onMessage(Message message) {
if (message.getType() == Message.MessageType.GAME && message.getMessage().contains("It's ready to add to the sapling.")) {
sapling.interact("Add-mulch");
}
}
It doesn't seem to be working.. :c
My bad everything seems to be a-okay! -
Thanks, Khal. I really can't thank you enough! Much love
-
1
-
-
So I created custombreakmanager and is there a way to track the time until the break/break duration on my mining script's paint? I've provided my custombreak manager/miner script's paint.
package Breaks;
import org.osbot.rs07.script.RandomEvent;
import org.osbot.rs07.script.RandomSolver;
import java.util.Random;
public class CustomBreakManager extends RandomSolver {
private long breakStartTime = 0;
private long breakDuration = 0;
private long remainingBreakTime = 0;
private long nextLongBreakTime = System.currentTimeMillis() + longBreakInterval();
private long nextMediumBreakTime = System.currentTimeMillis() + mediumBreakInterval();
private long nextSmallBreakTime = System.currentTimeMillis() + smallBreakInterval();
private boolean isBreaking = false;
private BreakType activeBreak = BreakType.NONE; // Track the active break type
Random random = new Random();
private enum BreakType {
NONE, SMALL, MEDIUM, LONG
}
public CustomBreakManager() {
super(RandomEvent.BREAK_MANAGER);
}
@Override
public boolean shouldActivate() {
long timeUntilSmallBreak = nextSmallBreakTime - System.currentTimeMillis();
long timeUntilMediumBreak = nextMediumBreakTime - System.currentTimeMillis();
long timeUntilLongBreak = nextLongBreakTime - System.currentTimeMillis();
return (timeUntilSmallBreak <= 0 && activeBreak == BreakType.NONE)
|| (timeUntilMediumBreak <= 0 && activeBreak == BreakType.NONE)
|| (timeUntilLongBreak <= 0 && activeBreak == BreakType.NONE);
}
@Override
public int onLoop() throws InterruptedException {
if (isBreaking) {
return 100; // Continue normal loop execution during the break
}
long nextSmallBreak = nextSmallBreakTime - System.currentTimeMillis();
long nextMediumBreak = nextMediumBreakTime - System.currentTimeMillis();
long nextLongBreak = nextLongBreakTime - System.currentTimeMillis();
if (nextSmallBreak <= 0 && activeBreak == BreakType.NONE) {
activeBreak = BreakType.SMALL;
startSmallBreak();
} else if (nextMediumBreak <= 0 && activeBreak == BreakType.NONE) {
activeBreak = BreakType.MEDIUM;
startMediumBreak();
} else if (nextLongBreak <= 0 && activeBreak == BreakType.NONE) {
activeBreak = BreakType.LONG;
startLongBreak();
}
return 1000; // Return any suitable value here
}
private long longBreakInterval() {
return (long) ((10 * 60 * 60 * 1000) + (Math.random() * (4 * 60 * 60 * 1000)));
}
private void startLongBreak() throws InterruptedException {
long breakDurationLONG = (long) ((8 * 60 * 60 * 1000) + (Math.random() * (2 * 60 * 60 * 1000)));
isBreaking = true;
int randomChance = random.nextInt(100) + 1;
if (randomChance <= 35) {
getLogoutTab().logOut();
sleep(breakDurationLONG);
} else {
sleep(breakDurationLONG);
}
isBreaking = false;
activeBreak = BreakType.NONE;
nextLongBreakTime = System.currentTimeMillis() + longBreakInterval();
}
private long mediumBreakInterval() {
return (long) ((40 * 60 * 1000) + (Math.random() * (30 * 60 * 1000)));
}
private void startMediumBreak() throws InterruptedException {
long breakDurationMED = (long) ((100 * 60 * 1000) + (Math.random() * (50 * 60 * 1000)));
isBreaking = true;
int randomChance = random.nextInt(100) + 1;
if (randomChance <= 35) {
getLogoutTab().logOut();
sleep(breakDurationMED);
} else {
sleep(breakDurationMED);
}
isBreaking = false;
activeBreak = BreakType.NONE;
nextMediumBreakTime = System.currentTimeMillis() + mediumBreakInterval();
}
public long getNextBreakTime() {
if (activeBreak == BreakType.NONE) {
return Math.min(nextSmallBreakTime, Math.min(nextMediumBreakTime, nextLongBreakTime));
} else {
return -1; // No break scheduled when active break is in progress
}
}
private long smallBreakInterval() {
return (long) ((20 * 60 * 1000) + (Math.random() * (14 * 60 * 1000)));
}
private void startSmallBreak() throws InterruptedException {
long breakDuration = (long) ((6 * 60 * 1000) + (Math.random() * (5 * 60 * 1000)));
isBreaking = true;
int randomChance = random.nextInt(100) + 1;
if (randomChance <= 35) {
getLogoutTab().logOut();
sleep(breakDuration);
} else {
sleep(breakDuration);
}
isBreaking = false;
activeBreak = BreakType.NONE;
nextSmallBreakTime = System.currentTimeMillis() + smallBreakInterval();
}
}
MINER SCRIPT BELOW
@Override
public void onStart() {
startTime = System.currentTimeMillis();
getExperienceTracker().start(Skill.MINING);
// Initialize the customBreakManager instance
CustomBreakManager breaker = new CustomBreakManager();
breaker.exchangeContext(getBot());
bot.getRandomExecutor().overrideOSBotRandom(breaker);
}@Override
public void onPaint(Graphics2D g) {
// Call super method to draw the default paint first
super.onPaint(g);
// Mouse X crosshair
Point mP = getMouse().getPosition();
g.setColor(Color.WHITE);
g.drawLine(mP.x - 5, mP.y + 5, mP.x + 5, mP.y - 5);
g.drawLine(mP.x + 5, mP.y + 5, mP.x - 5, mP.y - 5);
// Trails
while (!mousePath.isEmpty() && mousePath.peek().isUp())
mousePath.remove();
Point clientCursor = mouse.getPosition();
MousePathPoint mpp = new MousePathPoint(clientCursor.x, clientCursor.y, 500);
if (mousePath.isEmpty() || !mousePath.getLast().equals(mpp))
mousePath.add(mpp);
MousePathPoint lastPoint = null;
for (MousePathPoint a : mousePath) {
if (lastPoint != null) {
g.setColor(nextColor());
g.drawLine(a.x, a.y, lastPoint.x, lastPoint.y);
}
lastPoint = a;
}
// Get the runtime in milliseconds
long runtime = System.currentTimeMillis() - startTime;
// Format runtime as a string
String runtimeString = String.format("Runtime: %02d:%02d:%02d",
TimeUnit.MILLISECONDS.toHours(runtime),
TimeUnit.MILLISECONDS.toMinutes(runtime) % 60,
TimeUnit.MILLISECONDS.toSeconds(runtime) % 60);
// Set font and color for the runtime display
g.setFont(new Font("Arial", Font.PLAIN, 14));
g.setColor(Color.WHITE);
// Draw the background rectangle
Color bgColor = new Color(0, 0, 0, 140);
Color nick = new Color(0, 0, 0, 255);
int rectX = 6;
int rectY = 345;
int rectWidth = g.getFontMetrics().stringWidth(runtimeString) + 395;
int rectHeight = g.getFontMetrics().getHeight() + 117;
g.setColor(bgColor);
g.fillRect(rectX, rectY, rectWidth, rectHeight);
g.setColor(nick);
g.fillRect(rectX, rectY + rectHeight + 18, rectWidth, g.getFontMetrics().getHeight() + 3);
// Draw the runtime string on top of the background
g.setColor(Color.WHITE);
g.drawString(runtimeString, rectX + 4, rectY + 15);
// Percentages
double miningPercent = percentToNextLevel(Skill.MINING);
g.drawString(getTimeToLevel(), rectX + 344, rectY + 65);
g.drawString("Mining Level: " + getCurrentMiningLevel(), rectX + 404, rectY + 85);
g.drawString("Mining: " + miningPercent + "%", rectX + 421, rectY + 105);
// Retrieve the remaining time until the next break
long timeUntilBreak = customBreakManager.getNextBreakTime() - System.currentTimeMillis();
// Format the time until the next break as a string
String breakTimeString = String.format("Next Break: %02d:%02d:%02d",
TimeUnit.MILLISECONDS.toHours(timeUntilBreak),
TimeUnit.MILLISECONDS.toMinutes(timeUntilBreak) % 60,
TimeUnit.MILLISECONDS.toSeconds(timeUntilBreak) % 60);
// Set font and color for the break time display
g.setFont(new Font("Arial", Font.PLAIN, 14));
g.setColor(Color.WHITE);
// Draw the break time string on top of the background
g.drawString(breakTimeString, rectX + 4, rectY + 35);
}-
1
-
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
-
up
In game message listener trouble
in Scripting Help
Posted
Khaleesi, I'm very thankful for the tips and information! God bless.