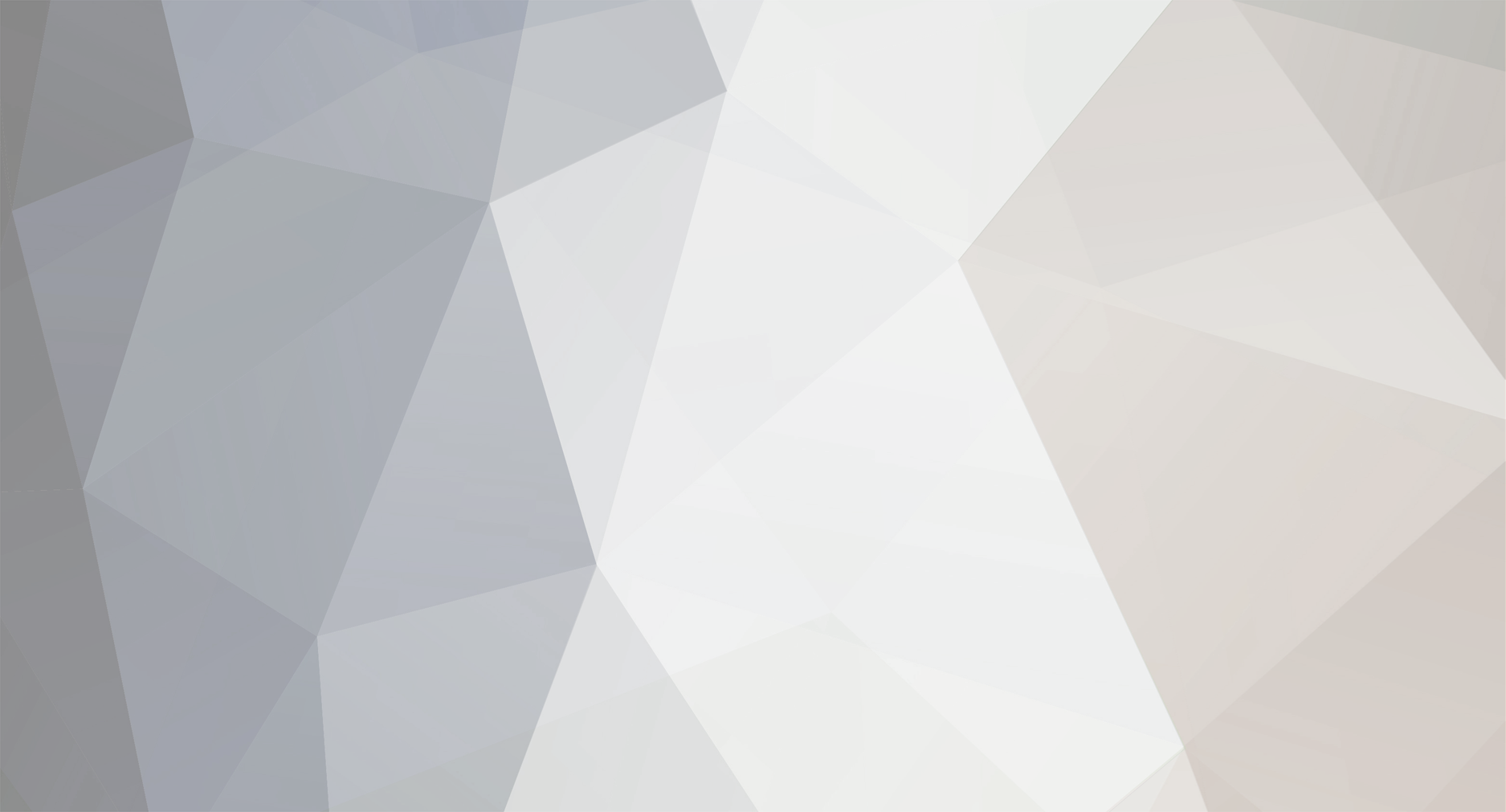
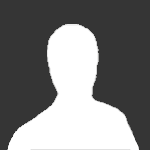
prolette
-
Posts
13 -
Joined
-
Last visited
-
Feedback
0%
Posts posted by prolette
-
-
Trick or Treat
-
Inshallah
6 hours ago, Maldesto said: -
Bump
-
On 10/19/2020 at 5:30 PM, Maldesto said:
@prolettehas won the giveaway!
Thanks so much man, i just logged in rn and i noticed I won! It might be the first time I've ever won a giveaway so this is kinda wild
-
1
-
-
This is amazing
-
6 minutes ago, Khaleesi said:
@proletteThat was probably my old tutorial for Osbot 1 client
public void onPaint(Graphics g)
Needs to be:
public void onPaint(Graphics2D g) {
It used to take a Graphics object but not it takes a graphics2D object
You can remove the following line aswell
Graphics2D gr = (Graphics2D)g;Thanks a lot for your help
9 minutes ago, Khaleesi said:@proletteThat was probably my old tutorial for Osbot 1 client
public void onPaint(Graphics g)
Needs to be:
public void onPaint(Graphics2D g) {
It used to take a Graphics object but not it takes a graphics2D object
You can remove the following line aswell
Graphics2D gr = (Graphics2D)g;Am I doing something wrong with the logs part cuz it doesn't change from 0?
-
I found my stupid mistake, sorry to bother you. I had to write Graphics2D at the end
-
1
-
-
Hello guys!
I followed a YouTube tutorial that shows you how to write a simple woodcutting script and I tried to follow all the steps correctly but I don't understand why the paint doesn't show up.
Maybe you guys could help a noob out.
package prolette.woodcutter; import org.osbot.rs07.api.Camera; import org.osbot.rs07.api.Client; import org.osbot.rs07.api.GrandExchange; import org.osbot.rs07.api.Inventory; import org.osbot.rs07.api.map.Area; import org.osbot.rs07.api.map.Position; import org.osbot.rs07.api.model.Entity; import org.osbot.rs07.api.ui.Skill; import org.osbot.rs07.script.MethodProvider; import org.osbot.rs07.script.Script; import org.osbot.rs07.script.ScriptManifest; import java.awt.*; @ScriptManifest(author = "prolette", name = "Woodcutter", info = "Chops trees near the GE and banks them.", version = 0.1, logo = "") public class Main extends Script { static Timer runTime; final String TREE_NAME = "Tree"; final Position BANKER_LOCATION = new Position(3162, 3490, 0); final Area GE = new Area(3147, 3507, 3182, 3471); final Area TREE_AREA = new Area( new int[][]{ { 3146, 3465 }, { 3147, 3457 }, { 3152, 3448 }, { 3159, 3449 }, { 3161, 3454 }, { 3162, 3459 }, { 3161, 3465 } } ); final int BANK_ID = 6528; int startExp; int startLvl; int logsChopped = 0; @Override public void onStart() throws InterruptedException { runTime = new Timer(0); startExp = getSkills().getExperience(Skill.WOODCUTTING); startLvl = getSkills().getVirtualLevel(Skill.WOODCUTTING); } @Override public void onExit() throws InterruptedException { } @Override public int onLoop() throws InterruptedException { if (!getInventory().isFull()) { if (TREE_AREA.contains(myPlayer())) { Entity tree = getObjects().closest(TREE_NAME); if (tree != null) { if (tree.isVisible()) { if (!myPlayer().isAnimating()) { if (!myPlayer().isMoving()) { tree.interact("Chop down"); sleep(random(700, 800)); } } } else { getCamera().toEntity(tree); } } }else { getWalking().webWalk(TREE_AREA); } }else { if (GE.contains(myPlayer())) { Entity banker = getNpcs().closest(BANK_ID); if (bank.isOpen()) { bank.depositAll(); }else { if (banker != null) { if (banker.isVisible()) { banker.interact("Bank"); sleep(random(700, 800)); }else { getCamera().toEntity(banker); } } } }else { getWalking().webWalk(BANKER_LOCATION); } } return 50; } public void onMessage(String message) { if (message.contains("You get some logs.")) { logsChopped++; } } public void onPaint(Graphics g) { Graphics2D gr = (Graphics2D)g; gr.setColor(Color.RED); gr.setFont(new Font("Arial",Font.PLAIN,10)); gr.drawString("Logs chopped: " + logsChopped, 12, 99); gr.drawString("Logs chopped/h: " + getPerHour(logsChopped), 12, 114); gr.drawString("Exp gained: " + (getSkills().getExperience(Skill.WOODCUTTING)- startExp), 12, 129); gr.drawString("Exp gained/h: " + getPerHour(getSkills().getExperience(Skill.WOODCUTTING)- startExp), 12, 144); gr.drawString("Lvls gained: " + (getSkills().getVirtualLevel(Skill.WOODCUTTING)- startLvl), 12, 159); gr.drawString("Lvls gained/h: " + getPerHour(getSkills().getVirtualLevel(Skill.WOODCUTTING)- startLvl), 12, 174); } public static int getPerHour(int value) { if (runTime != null && runTime.getElapsed() > 0) { return (int) (value * 3600000d / runTime.getElapsed()); }else return 0; } public static long getPerHour(long value) { if (runTime != null && runTime.getElapsed() > 0) { return (long) (value * 3600000d / runTime.getElapsed()); }else return 0; } public class Timer { private long period; private long start; public Timer(long period) { this.period = period; start = System.currentTimeMillis(); } public long getElapsed() { return System.currentTimeMillis() - start; } public long getRemaining() { return period - getElapsed(); } public boolean isRunning() { return getElapsed() <= period; } public void reset() { start = System.currentTimeMillis(); } public void stop() { period = 0; } public String format(long milliSeconds) { long secs = milliSeconds / 1000L; return String.format("%02d:%02d:%02d", new Object[] { Long.valueOf(secs / 3600L), Long.valueOf((secs % 3600L) / 60L), Long.valueOf(secs % 60L) }); } } }
-
1
-
-
Could I have a trial, pretty please? I am kinda low on money rn and I wanna know if it is worth my investment.
Christmas Script giveaways PARTIAL WINNER ANNOUNCED
in News & Announcements
Posted
1. OSRS Script Factory Pro Edition
2. Perfect Runecrafting
3. KO Crazy Archy
4. Khal Tempoross
5. Progamerz Abyss Runecrafter