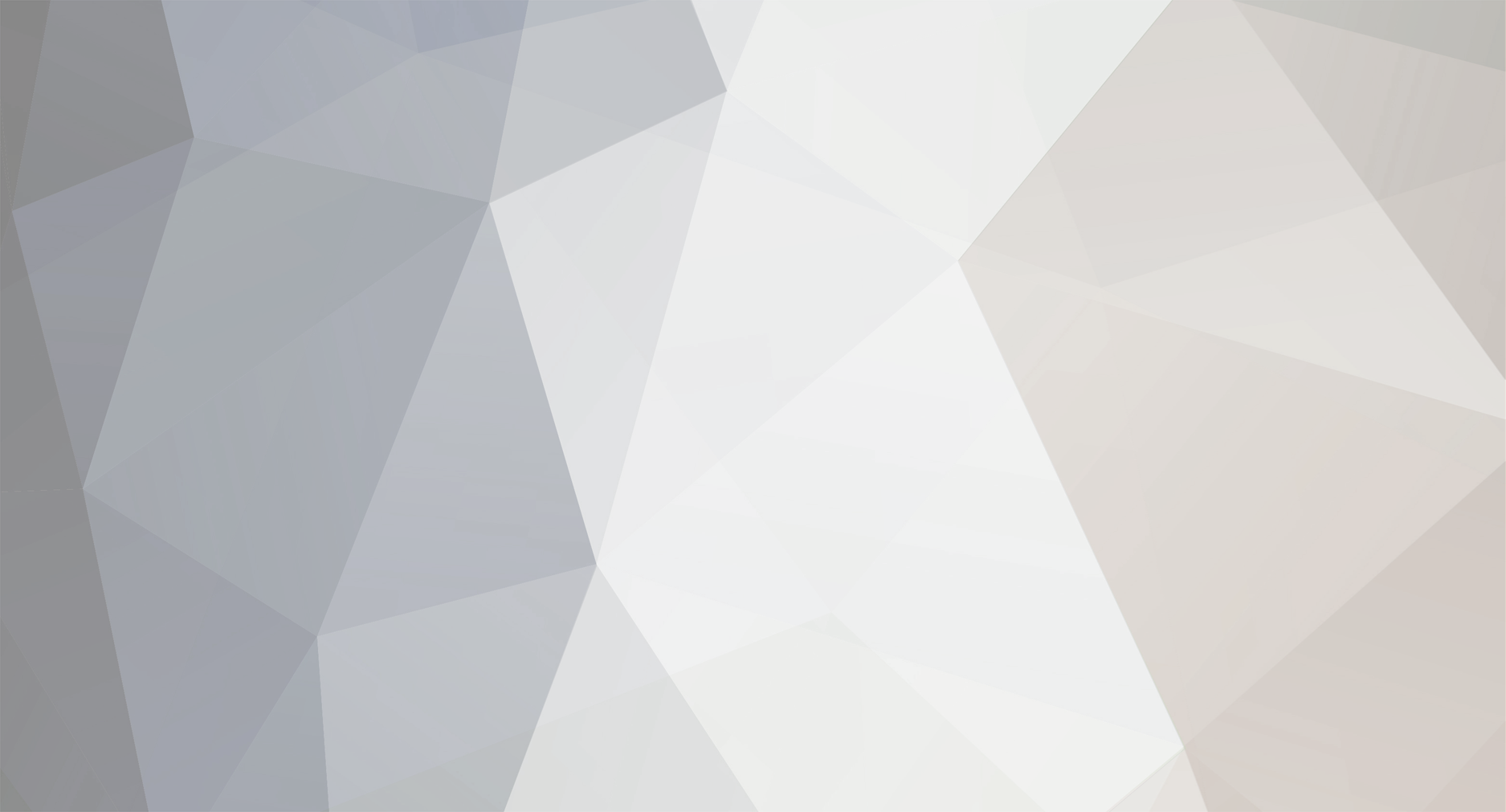
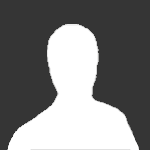
Psvxe
-
Posts
235 -
Joined
-
Last visited
-
Feedback
100%
Posts posted by Psvxe
-
-
What I was wondering aswell. On another note though, I tried it and it works for me.
Your method would make somewhat sense if you were making an api, seeing as you didnt pass a variable im assuming thats not the case, i'd use the snippet LoudPacks gave.
If you are using it to make a universal method, try this.
void dropAll(String itemName) { if(!myPlayer().isAnimating()) { for(Item i : getInventory().getItems()) { if(i.getName() == itemName) i.interact("Drop"); //Would typically be your own drop method here } } }
Why would check within the method if player is animating and only drop if it isn't?
Doesn't really make sense to me.
-
As a S2 you should remember those methods and not rely on the API docs.
As a S2 you should understand he's asking why they're gone rather than what they do.
-
4
-
-
Make the script stop itself as soon as it starts, best anti-ban imo
No, Don't bot at all. Stop playing the game. No way you would get banned.
-
I guess you could use a second thread if you willing to do something different than the main job of your script. Don't take it for granted though. Never did that shit since I never got banned when I'd use a script without anti-ban features.
-
I got it!
If you guys are interested, i'm willing to translate everything to english and publish it here. Could help some people who are learning basic Java.
for(int i=0; i<questions.length; i++) { group[i] = new ToggleGroup(); Label label = new Label((i+1) + questions[i]); grid.add(label, 0, i); agree[i] = new RadioButton("Agreed"); agree[i].setToggleGroup(group[i]); grid.add(agree[i], 1, i); disagree[i] = new RadioButton("Disagree"); disagree[i].setToggleGroup(group[i]); grid.add(disagree[i], 2, i); no_opinion[i] = new RadioButton("No opinion"); no_opinion[i].setToggleGroup(group[i]); grid.add(no_opinion[i], 3, i); }
-
Use knives.
-
Obviously not :E, " " is the HTML version
LOL at those scripters saying to use that.
-
Print the bytes
Edited the post above me.
\\u00A0
did eventually worked for me to replace the non-breaking space. nbsp; didn't do the job. -
Actually, I did some debugging myself for fun and I figured out that some names do have normal spaces, or more likely, they don't seem to be non-breaking spaces.
[INFO][Bot #1][03/30 09:45:02 AM]: Player : Lil Slurp = Lil Slurp [INFO][Bot #1][03/30 09:45:02 AM]: Player : king ammar x = king ammar x [INFO][Bot #1][03/30 09:45:02 AM]: Player : 66�Atlas�998 = 66�Atlas�998 [INFO][Bot #1][03/30 09:45:02 AM]: Player : Item�Robber = Item�Robber
Something is different, no clue what.EDIT:
Alright.
Itz�PRADA Itz PRADA [INFO][Bot #1][03/30 10:01:06 AM]: Player : Stanklin Stanklin [INFO][Bot #1][03/30 10:01:06 AM]: Player : EpezuWnBnoob EpezuWnBnoob [INFO][Bot #1][03/30 10:01:06 AM]: Player : NatusVincere NatusVincere [INFO][Bot #1][03/30 10:01:06 AM]: Player : JollyJJoker JollyJJoker [INFO][Bot #1][03/30 10:01:06 AM]: Player : Kitten_Dance Kitten_Dance [INFO][Bot #1][03/30 10:01:06 AM]: Player : king ammar x king ammar x [INFO][Bot #1][03/30 10:01:06 AM]: Player : Inneva Inneva [INFO][Bot #1][03/30 10:01:06 AM]: Player : Erza Kagura Erza Kagura [INFO][Bot #1][03/30 10:01:06 AM]: Player : SG Editz SG Editz [INFO][Bot #1][03/30 10:01:06 AM]: Player : Lil Slurp Lil Slurp [INFO][Bot #1][03/30 10:01:06 AM]: Player : mag3king6424 mag3king6424 [INFO][Bot #1][03/30 10:01:06 AM]: Player : 39�Bork�Roc 39 Bork Roc [INFO][Bot #1][03/30 10:01:06 AM]: Player : 54ancient428 54ancient428
Done with the following codefor (Player player : ctx.players.getAll()) { if (player != null) { String orginal_name = player.getName().replaceAll("\\u00A0", " "); ctx.log("Player : " + player.getName() + " " + orginal_name); } }
\\u00A0
Good luck. -
Search before starting new threads.
http://osbot.org/forum/topic/95180-myplayergetnameequalsbla-bla/
-
Send me a pm with your Skype. I'll help you out.. Made the quest already.
-
1
-
-
I guess I am like the only one that did learn java trough just writing programs and googling around if I couldn't get the answer here..
I advice you to follow some study though. Good luck
-
Someone is going strong on releasing snippets. Nice release.
-
I did create a similar framework.
I'm not quite sure if I understand you right however.
Do you mean you want to add / pause / remove tasks / nodes on conditions from any other task you're running at the moment?
If so, I can help you.
-
uhh not sure about this but wasn't a fishing spot an npc?
So it'd be NPC fishSpot = getNpcs().closest("Fishing spot")
check however im not entirely sure lol
You´re correct.
-
Game message or check if door has option. If not you're good to go.
-
Nice, I wrote this a while ago
definitely more succinct to use setLocationRelativeTo(null)
Still a good read for beginners who want to make a GUI
-
Thanks for the help!
Would you suggest I define my WebWalkEvents at the top of my script as public (where i have them in the above source) or should i define them right before I call them?
You should define it before you call them, yes. I have my own framework so I did it different. However, don't do it like the guy above me said. That sucks.
Send me a pm if you need help.
-
// Declare two constants for width and height of the GUI final int GUI_WIDTH = 350, GUI_HEIGHT = 75; // Get the size of the screen Dimension screenSize = Toolkit.getDefaultToolkit().getScreenSize(); // Calculating x and y coordinates final int gX = (int) (screenSize.getWidth() / 2) - (GUI_WIDTH / 2); final int gY = (int) (screenSize.getHeight() / 2) - (GUI_HEIGHT / 2);
Or to set a size to exactly your likinggui#setSize(int width, int heigth) //size of the gui; gui#setLocationRelativeTo(null); //centers the gui
Nice guide, will help the noobs. good job!-
1
-
-
Shit code, didn't know how to do it too first and this was my first try on it. Here you go. I dumped all item prices using this
public static TreeMap<Integer, Integer> dump_map = new TreeMap<Integer, Integer>(); public static HashMap<Integer, Integer> current_item_map = new HashMap<Integer, Integer>(); public static void main(String[] args) throws IOException { toTreeMap(); } /** * * @param itemId * @return price of the item */ public static int getPrice(int itemId) { try { URL url = new URL("https://api.rsbuddy.com/grandExchange?a=guidePrice&i=" + itemId); try (BufferedReader reader = new BufferedReader(new InputStreamReader(url.openStream()))) { String line = reader.readLine(); return line == null ? -1 : Integer.parseInt(line.substring(11, line.indexOf(','))); } catch (IOException e) { e.printStackTrace(); } } catch (MalformedURLException e) { e.printStackTrace(); } return -1; } /** * * @param val * @throws IOException * */ public static void getItems() throws IOException { int count = 0; try { FileReader in = new FileReader(System.getProperty("user.home") + "/Dump.txt"); BufferedReader br = new BufferedReader(in); String line = br.readLine(); while (line != null) { System.out.println(line); count++; line = br.readLine(); } in.close(); } catch (IOException e) { e.printStackTrace(); } } /** * * @param id * @return priceFromFile */ public static Integer getPriceFromFile(int id) { try { FileReader in = new FileReader(System.getProperty("user.home") + "/Dump.txt"); BufferedReader br = new BufferedReader(in); String line = br.readLine(); while (line != null && !line.contains(Integer.toString(id))) { line = br.readLine(); } String content = line.substring(line.lastIndexOf(' ') + 1); in.close(); System.out.println(id + " >" + Integer.parseInt(content) + "<"); return line.lastIndexOf(Integer.parseInt(content)); } catch (IOException e) { e.printStackTrace(); } return -1; } /** * * @param val * @return Text file with items from @param. */ public static void grabItems(int val) { for (int i = 1; i < 13193; i++) { try { File file = new File(System.getProperty("user.home") + "/Dump.txt"); if (!file.exists()) { file.createNewFile(); } FileWriter fw = new FileWriter(file.getAbsoluteFile(), true); BufferedWriter bw = new BufferedWriter(fw); bw.newLine(); while (getPrice(i) < val) { i++; } System.out.println(i + " Value: " + getPrice(i)); bw.write(i + " " + getPrice(i)); bw.flush(); bw.close(); } catch (IOException e) { e.printStackTrace(); } } } /** * @return all items into a treeMap. */ public static void toTreeMap() { String filePath = System.getProperty("user.home") + "/Dump.txt"; try { String line; BufferedReader br = new BufferedReader(new FileReader(filePath)); while ((line = br.readLine()) != null) { String[] parts = line.split(" ", 2); if (parts.length >= 2) { int key = Integer.parseInt(parts[0]); int value = Integer.parseInt(parts[1]); dump_map.put(key, value); System.out.println(dump_map.put(key, value)); } else { System.out.println("Ingoring line."); } } br.close(); } catch (IOException e) { e.printStackTrace(); } } public static void getKeys() { toTreeMap(); Set<Integer> keys = dump_map.keySet(); System.out.println(keys); } public static <K, V extends Comparable<? super V>> SortedSet<Map.Entry<K, V>> entriesSortedByValues(Map<K, V> map) { SortedSet<Map.Entry<K, V>> sortedEntries = new TreeSet<Map.Entry<K, V>>(new Comparator<Map.Entry<K, V>>() { @Override public int compare(Map.Entry<K, V> e1, Map.Entry<K, V> e2) { int res = e1.getValue().compareTo(e2.getValue()); return res != 0 ? res : 1; } }); sortedEntries.addAll(map.entrySet()); return sortedEntries; } public static void getHighestValue() { toTreeMap(); for (Entry<Integer, Integer> entry : entriesSortedByValues(dump_map)) { System.out.println(entry.getKey() + ":" + entry.getValue()); } }
EDIT: yeah. It's shit but works. If I were you I wouldn't use it in my script. -
Well if you do
WebWalkEvent evt = new WebWalkEvent(Manager.routeFinder, new Position(1500, 1700, 0), new Position(1000, 1000, 0), new Position(2222,2222,0);
it will stop at any of the destinations you specified, (1500,1700) (1000,1000) and (2222,2222). If you want to walk from A to B passing some waypoints you could just execute multiple WWevents, one for each waypoint right? Another option might be to change the costs for waypoints, similar to how it's done for avoidproxy which basically sets the cost to 999999 for specific nodes, you could try to set it to a small or negative value. Not sure if this would work tho...Yeah, I just figured that out since I found out the javadocs are back online. haha
Using different WebWalkEvent for just walking to one end-coordinate feels messy.
I could try the last option you gave me though. No clue if that will work.
-
There's api.webwalk.impl.AvoidProxy which you can use to avoid certain areas. MGI posted this as an example:
INodeRouteFinder finder = INodeRouteFinder.createAdvanced(); for (INodeScript script : finder.scripts()) { finder.remove(script); finder.add(new AvoidProxy(script, true, BLOCK_AREA_1, BLOCK_AREA_2)); }
Thanks, this will solve the current issue for now I have!
However, I still would like to know if my question is possible. Since I had to bypass a certain situation a while back because of the above stated question, too. I can't use areas to solve that.
-
Fair enough:
Solved x)
haha, Great!
-
Searched around but couldn't find the right answer. Perhaps I just didn't notice it but I think my question isn't asked at all.
Please let me explain the problem I have.
I need to walk to a coordinate. The fastest route is trough a dangerous zone (red dots) and the safe route (green dots) is a bit longer.
Now it will simply walk to the end-coordinate (purple dot) trough the dangerous zone and I don't want that.
What I did try is to something like
WebWalkEvent evt = new WebWalkEvent(Manager.routeFinder, new Position(1500, 1700, 0), new Position(1000, 1000, 0), new Position(2222,2222,0);
However it wouldn't walk any further than when it reached the first coordinate I set (1500, 1700, 0).Any solutions without setting pre-defined paths?
how and when to use filters with npc's
in Scripting Help
Posted · Edited by Psvxe
I get NPE´s to when doing